利用三张不同角度拍摄的特定场景图像,实现图像拼接,要求用C++和OPENCV编程实现,使用stitching函数进行图像拼接
时间: 2024-02-09 08:12:28 浏览: 28
好的,我理解了你的问题。要实现图像拼接,你需要使用C++和OpenCV编程,并使用OpenCV中的stitching函数。以下是实现图像拼接的步骤:
1. 导入OpenCV库,并加载需要拼接的图像。
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main() {
// 加载需要拼接的图像
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
Mat img3 = imread("img3.jpg");
if (img1.empty() || img2.empty() || img3.empty()) {
cout << "无法加载图像文件!" << endl;
return -1;
}
```
2. 将需要拼接的图像存储到一个vector中。
```c++
// 将所有图像存储到一个vector中
vector<Mat> imgs;
imgs.push_back(img1);
imgs.push_back(img2);
imgs.push_back(img3);
```
3. 创建stitcher对象,并设置相关参数。
```c++
// 创建stitcher对象
Ptr<Stitcher> stitcher = Stitcher::createDefault();
// 设置stitcher对象的参数
Stitcher::Mode mode = Stitcher::PANORAMA; // 设置拼接模式为全景
stitcher->setMode(mode);
```
4. 使用stitching函数进行图像拼接,并输出拼接结果。
```c++
// 拼接图像
Mat pano;
Stitcher::Status status = stitcher->stitch(imgs, pano);
// 判断拼接是否成功
if (status != Stitcher::OK) {
cout << "图像拼接失败!" << endl;
return -1;
}
// 输出拼接结果
imwrite("pano.jpg", pano);
imshow("拼接结果", pano);
waitKey(0);
return 0;
}
```
完整的代码如下所示:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace std;
using namespace cv;
int main() {
// 加载需要拼接的图像
Mat img1 = imread("img1.jpg");
Mat img2 = imread("img2.jpg");
Mat img3 = imread("img3.jpg");
if (img1.empty() || img2.empty() || img3.empty()) {
cout << "无法加载图像文件!" << endl;
return -1;
}
// 将所有图像存储到一个vector中
vector<Mat> imgs;
imgs.push_back(img1);
imgs.push_back(img2);
imgs.push_back(img3);
// 创建stitcher对象
Ptr<Stitcher> stitcher = Stitcher::createDefault();
// 设置stitcher对象的参数
Stitcher::Mode mode = Stitcher::PANORAMA; // 设置拼接模式为全景
stitcher->setMode(mode);
// 拼接图像
Mat pano;
Stitcher::Status status = stitcher->stitch(imgs, pano);
// 判断拼接是否成功
if (status != Stitcher::OK) {
cout << "图像拼接失败!" << endl;
return -1;
}
// 输出拼接结果
imwrite("pano.jpg", pano);
imshow("拼接结果", pano);
waitKey(0);
return 0;
}
```
希望这个回答能够帮助到你!
相关推荐
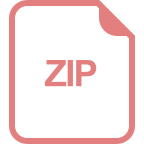
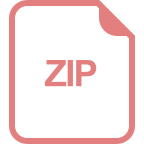












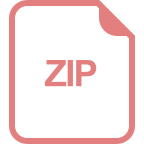