Socket client = null; ObjectOutputStream oos = null; ObjectInputStream ois = null; try { client = new Socket(InetAddress.getByName("192.168.3.18"), 6666); //服务器地址 OutputStream out = client.getOutputStream(); oos = new ObjectOutputStream(out); oos.writeUTF("你好,服务器!"); oos.flush(); InputStream in = client.getInputStream(); ois = new ObjectInputStream(in); String message = ois.readUTF(); System.out.println(message); } catch (IOException e) { e.printStackTrace(); } finally { try { ois.close(); oos.close(); client.close(); } catch (IOException e) { e.printStackTrace(); } } 解释一下这段代码
时间: 2024-04-17 10:23:51 浏览: 9
这段代码是一个简单的Socket客户端实现。它通过创建一个Socket对象连接到指定的服务器地址和端口(这里是192.168.3.18:6666)。然后,使用Socket的getOutputStream()方法获取输出流,再包装成ObjectOutputStream对象oos。接着,通过oos的writeUTF()方法发送一条消息给服务器,并使用flush()方法强制刷新缓冲区,确保消息被发送。
然后,使用Socket的getInputStream()方法获取输入流,再包装成ObjectInputStream对象ois。通过ois的readUTF()方法从输入流中读取服务器发送的消息,并将其赋值给变量message。最后,将消息打印出来。
无论是否发生异常,最后都会执行finally块中的代码,关闭ois、oos和Socket对象。
需要注意的是,该代码只是一个简单示例,并没有处理异常情况和多线程。在实际应用中,应该根据具体需求进行适当的异常处理和线程管理。
相关问题
ServerSocket server = null; Socket client = null; ObjectInputStream ois = null; ObjectOutput oos = null; try { server = new ServerSocket(6666); client = server.accept(); InputStream in = client.getInputStream(); ois = new ObjectInputStream(in); String message = ois.readUTF(); System.out.println(message); OutputStream out = client.getOutputStream(); oos = new ObjectOutputStream(out); oos.writeUTF("你好,客户端!"); oos.flush(); } catch (IOException e) { e.printStackTrace(); } finally { try { oos.close(); ois.close(); client.close(); server.close(); } catch (IOException e) { e.printStackTrace(); } } 解释这段代码每行的意思
这段代码是一个简单的Socket服务器实现。让我们逐行解释每一行的意思:
1. `ServerSocket server = null;` - 声明了一个ServerSocket对象变量`server`,初始化为null。
2. `Socket client = null;` - 声明了一个Socket对象变量`client`,初始化为null。
3. `ObjectInputStream ois = null;` - 声明了一个ObjectInputStream对象变量`ois`,初始化为null。
4. `ObjectOutput oos = null;` - 声明了一个ObjectOutput对象变量`oos`,初始化为null。
5. `server = new ServerSocket(6666);` - 创建一个ServerSocket对象,并绑定到指定的端口号6666。
6. `client = server.accept();` - 等待客户端的连接请求,并接受连接。一旦有客户端连接成功,将返回一个Socket对象,该对象表示与客户端的通信通道。
7. `InputStream in = client.getInputStream();` - 从Socket对象中获取输入流,用于接收来自客户端的数据。
8. `ois = new ObjectInputStream(in);` - 使用输入流创建一个ObjectInputStream对象`ois`,用于从输入流中读取对象。
9. `String message = ois.readUTF();` - 从输入流中读取一个UTF字符串,即客户端发送的消息,并将其赋值给变量`message`。
10. `System.out.println(message);` - 打印接收到的消息。
11. `OutputStream out = client.getOutputStream();` - 从Socket对象中获取输出流,用于向客户端发送数据。
12. `oos = new ObjectOutputStream(out);` - 使用输出流创建一个ObjectOutputStream对象`oos`,用于向输出流中写入对象。
13. `oos.writeUTF("你好,客户端!");` - 向输出流中写入一个UTF字符串,即向客户端发送消息。
14. `oos.flush();` - 强制刷新输出流,确保消息被发送。
在异常处理部分,如果发生异常,将打印异常栈轨迹信息。
最后,在finally块中,关闭oos、ois、client和server对象的输入输出流,并关闭client和server的Socket连接。
请解释:socket = new Socket(InetAddress.getByName(ipText.getText()), Integer.parseInt(portText.getText())); //得到ObjectOutputStream ObjectOutputStream oos = new ObjectOutputStream(socket.getOutputStream()); //发送user对象 oos.writeObject(user); //读取服务端回复 ObjectInputStream ois = new ObjectInputStream(socket.getInputStream());
这段代码是用于建立客户端与服务器之间的 socket 连接,并通过 socket 连接传输 user 对象的。具体来说,代码的执行过程如下:
1. 通过 InetAddress.getByName(ipText.getText()) 获取指定 IP 地址的 InetAddress 对象。
2. 通过 Integer.parseInt(portText.getText()) 获取指定端口号,并使用 InetAddress 和端口号创建 Socket 对象。
3. 通过 socket.getOutputStream() 获取输出流,再通过 ObjectOutputStream 将 user 对象写入输出流中,发送给服务器。
4. 通过 socket.getInputStream() 获取输入流,再通过 ObjectInputStream 读取服务器返回的数据。
这段代码的主要作用是实现客户端与服务器之间的通信,通过 socket 连接来传输数据。其中,ObjectOutputStream 和 ObjectInputStream 分别用于将对象写入输出流和从输入流中读取对象。通过这种方式,客户端与服务端可以传输各种类型的数据,包括自定义的对象。
相关推荐
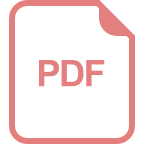
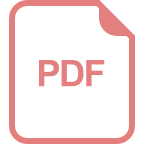
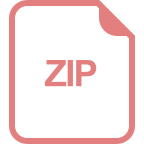













