用c++实现对线性表的删除数据元素,查找数据元素,增加数据元素
时间: 2024-09-19 11:16:12 浏览: 35
在 C++ 中,我们可以使用多种数据结构如数组、链表或动态容器(如`std::vector`或`std::list`)来实现线性表的操作。这里我们分别介绍如何在链表和数组这两种常见数据结构上进行插入、删除和查找操作:
**1. 链表(Linked List)**
- **删除数据元素(Delete)**: 对于单链表,可以遍历找到要删除的节点,然后调整前一个节点的 `next` 指针指向下一个节点;对于双向链表,需要同时更新前向和后向指针。
```cpp
struct Node {
int data;
Node* next;
};
void deleteNode(Node*& head, int value) {
if (head == nullptr || head->data == value) {
// 如果头结点就是要删除的,特殊处理
head = head->next;
} else {
Node* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr)
current->next = current->next->next;
}
}
```
- **查找数据元素(Find)**: 使用迭代或递归遍历链表直到找到目标值或遍历完。
```cpp
bool search(Node* head, int value) {
Node* current = head;
while (current != nullptr) {
if (current->data == value)
return true;
current = current->next;
}
return false;
}
- **增加数据元素(Insert)**: 在适当位置创建新节点并连接到链表。
```cpp
void insertNode(Node*& head, int value, int position) {
if (position < 0)
return;
Node* newNode = new Node{value};
if (position == 0) {
newNode->next = head;
head = newNode;
} else {
Node* current = head;
for (int i = 0; i < position - 1 && current != nullptr; ++i)
current = current->next;
if (current)
current->next = newNode;
newNode->next = current->next;
}
}
```
**2. 数组(Array)**
- **删除(Delete)**: 在动态数组中通常通过移动其他元素来替换删除元素的位置,然后缩小数组大小。
```cpp
std::vector<int> arr;
// 删除元素操作...
void removeElement(int index) {
std::copy(arr.begin() + index + 1, arr.end(), arr.begin() + index);
arr.pop_back();
}
```
- **查找(Find)**: 直接访问数组元素,如果找到则返回索引,否则返回 `std::string::npos` 或 `-1`。
```cpp
size_t findElement(const std::vector<int>& arr, int value) {
return std::find(arr.begin(), arr.end(), value) - arr.begin();
}
- **增加(Insert)**: 插入操作一般涉及到动态扩容,这里仅演示基本插入:
```cpp
void insertElement(std::vector<int>& arr, int value, size_t position) {
if (position > arr.size()) {
arr.resize(arr.size() * 2); // 扩容一倍
}
arr.insert(arr.begin() + position, value);
}
```
阅读全文
相关推荐
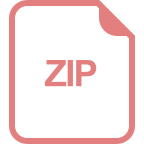
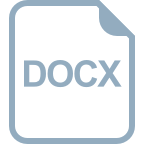
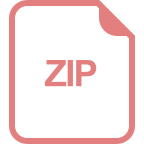
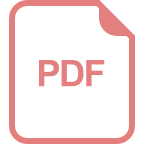
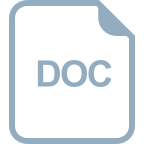
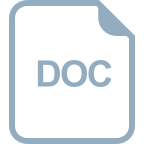
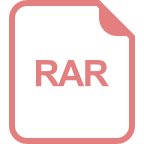
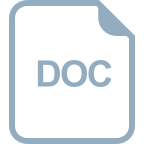
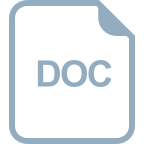
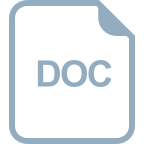
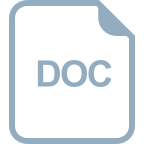
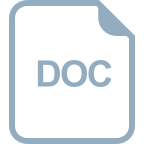
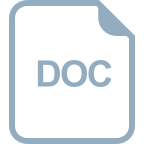
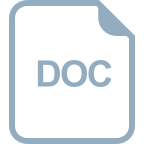
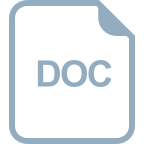
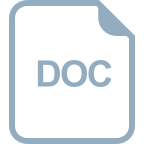
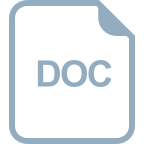
