dev c++写贪吃蛇
时间: 2024-10-17 15:01:02 浏览: 42
在Dev-C++中编写贪吃蛇游戏是一个常见的计算机科学练习项目,它通常涉及到基本的图形界面编程、事件处理和算法设计。以下是创建一个简单版本的步骤:
1. **环境设置**:
- 安装Dev-C++并配置好mingw-w64编译器。
- 创建一个新的Windows Console Application项目。
2. **基本框架**:
- 使用C++头文件如`windows.h`引入必要的库,以支持窗口和图形操作。
- 设计游戏区域(屏幕)大小,并在主循环中更新和绘制屏幕内容。
3. **贪吃蛇角色**:
- 创建一个 Snake 类,包含位置、长度和方向等属性。
- 创建 Food 类,随机在地图上生成位置。
- 当蛇吃到食物时,增加它的长度,同时在另一个随机位置生成新的食物。
5. **用户输入和控制**:
- 添加一个事件处理器函数,监听键盘输入,根据按键调整蛇的方向。
6. **循环和控制流程**:
- 在主循环中,检查碰撞(包括蛇身与边界、蛇身自身或食物),并根据结果更新状态。
- 渲染新画面,然后等待下一个事件。
7. **保存和加载游戏状态**:
(可选)为了使得游戏暂停或继续更方便,可以实现简单的游戏存档和读取功能。
相关问题
用DEV c++写贪吃蛇
当然可以使用Dev C++来编写贪吃蛇游戏!下面是一个简单的示例代码:
```c
#include <conio.h>
#include <windows.h>
bool gameOver;
const int width = 20;
const int height = 20;
int x, y, fruitX, fruitY, score;
int tailX[100], tailY[100];
int nTail;
enum eDirection { STOP = 0, LEFT, RIGHT, UP, DOWN };
eDirection dir;
void Setup()
{
gameOver = false;
dir = STOP;
x = width / 2;
y = height / 2;
fruitX = rand() % width;
fruitY = rand() % height;
score = 0;
}
void Draw()
{
system("cls");
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
if (j == 0)
cout << "#";
if (i == y && j == x)
cout << "O";
else if (i == fruitY && j == fruitX)
cout << "F";
else
{
bool printTail = false;
for (int k = 0; k < nTail; k++)
{
if (tailX[k] == j && tailY[k] == i)
{
cout << "o";
printTail = true;
}
}
if (!printTail)
cout << " ";
}
if (j == width - 1)
cout << "#";
}
cout << endl;
}
for (int i = 0; i < width + 2; i++)
cout << "#";
cout << endl;
cout << "Score:" << score << endl;
}
void Input()
{
if (_kbhit())
{
switch (_getch())
{
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
gameOver = true;
break;
}
}
}
void Logic()
{
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = x;
tailY[0] = y;
for (int i = 1; i < nTail; i++)
{
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
switch (dir)
{
case LEFT:
x--;
break;
case RIGHT:
x++;
break;
case UP:
y--;
break;
case DOWN:
y++;
break;
default:
break;
}
if (x >= width)
x = 0;
else if (x < 0)
x = width - 1;
if (y >= height)
y = 0;
else if (y < 0)
y = height - 1;
for (int i = 0; i < nTail; i++)
{
if (tailX[i] == x && tailY[i] == y)
gameOver = true;
}
if (x == fruitX && y == fruitY)
{
score += 10;
fruitX = rand() % width;
fruitY = rand() % height;
nTail++;
}
}
int main()
{
Setup();
while (!gameOver)
{
Draw();
Input();
Logic();
Sleep(10); // 控制游戏速度,单位毫秒
}
return 0;
}
```
这是一个简单的贪吃蛇游戏示例,使用了Dev C++作为开发环境。代码中使用了Windows.h头文件中的一些函数来处理控制台窗口的输入输出。你可以在Dev C++中创建一个新的项目,将代码复制到源文件中进行编译运行。
用devc++制作贪吃蛇游戏
好的,我可以为您提供一些基础代码,您可以在Dev-C++中进行修改和调试。
```c++
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define MAX_X 50 // 地图的宽度
#define MAX_Y 20 // 地图的高度
// 定义一个结构体表示蛇的每个节点
struct Node {
int x; // 节点的横坐标
int y; // 节点的纵坐标
struct Node *next; // 指向下一个节点的指针
};
// 定义一个结构体表示食物
struct Food {
int x; // 食物的横坐标
int y; // 食物的纵坐标
};
// 定义一个枚举类型表示蛇的运动方向
enum Direct {
UP,
DOWN,
LEFT,
RIGHT
};
int length = 3; // 蛇的长度
struct Node *head; // 蛇头指针
struct Node *tail; // 蛇尾指针
struct Food food; // 食物
enum Direct direct; // 蛇的运动方向
int score = 0; // 分数
// 初始化地图
void init() {
// 隐藏光标
CONSOLE_CURSOR_INFO cursor_info = { 1, 0 };
SetConsoleCursorInfo(GetStdHandle(STD_OUTPUT_HANDLE), &cursor_info);
// 随机生成食物的位置
srand((unsigned int)time(NULL));
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
// 初始化蛇的位置
head = (struct Node *)malloc(sizeof(struct Node));
head->x = MAX_X / 2;
head->y = MAX_Y / 2;
head->next = NULL;
tail = head;
for (int i = 1; i < length; i++) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->x = head->x + i;
node->y = head->y;
node->next = NULL;
tail->next = node;
tail = node;
}
// 初始化蛇的运动方向
direct = RIGHT;
}
// 在屏幕上画出地图
void drawMap() {
system("cls"); // 清空屏幕
for (int i = 0; i <= MAX_X + 1; i++) {
printf("#");
}
printf("\n");
for (int i = 0; i < MAX_Y; i++) {
printf("#");
for (int j = 0; j < MAX_X; j++) {
if (i == head->y && j == head->x) { // 如果是蛇头
printf("@");
} else if (i == food.y && j == food.x) { // 如果是食物
printf("*");
} else {
bool flag = false;
struct Node *p = head->next;
while (p != NULL) { // 如果是蛇身
if (p->x == j && p->y == i) {
printf("#");
flag = true;
break;
}
p = p->next;
}
if (!flag) {
printf(" ");
}
}
}
printf("#");
printf("\n");
}
for (int i = 0; i <= MAX_X + 1; i++) {
printf("#");
}
printf("\n");
printf("Score: %d\n", score);
}
// 判断蛇是否吃到了食物
bool isEatFood() {
return head->x == food.x && head->y == food.y;
}
// 在屏幕上画出食物
void drawFood() {
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
struct Node *p = head;
while (p != NULL) {
if (food.x == p->x && food.y == p->y) { // 如果食物与蛇身重合,重新生成
food.x = rand() % MAX_X;
food.y = rand() % MAX_Y;
p = head;
continue;
}
p = p->next;
}
gotoxy(food.x + 1, food.y + 1);
printf("*");
}
// 蛇的运动
void move() {
struct Node *newHead = (struct Node *)malloc(sizeof(struct Node));
newHead->x = head->x;
newHead->y = head->y;
switch (direct) {
case UP:
newHead->y--;
break;
case DOWN:
newHead->y++;
break;
case LEFT:
newHead->x--;
break;
case RIGHT:
newHead->x++;
break;
}
newHead->next = head;
head = newHead;
if (!isEatFood()) {
struct Node *p = head;
while (p->next->next != NULL) {
p = p->next;
}
tail = p;
free(p->next);
p->next = NULL;
} else {
length++;
score++;
drawFood();
}
}
// 判断游戏是否结束
bool isGameOver() {
if (head->x < 0 || head->x >= MAX_X || head->y < 0 || head->y >= MAX_Y) { // 是否撞墙
return true;
}
struct Node *p = head->next;
while (p != NULL) { // 是否撞到自己的身体
if (head->x == p->x && head->y == p->y) {
return true;
}
p = p->next;
}
return false;
}
// 控制蛇的运动方向
void control() {
if (_kbhit()) {
char ch = _getch();
if (ch == 'w' || ch == 'W') {
if (direct != DOWN) {
direct = UP;
}
} else if (ch == 's' || ch == 'S') {
if (direct != UP) {
direct = DOWN;
}
} else if (ch == 'a' || ch == 'A') {
if (direct != RIGHT) {
direct = LEFT;
}
} else if (ch == 'd' || ch == 'D') {
if (direct != LEFT) {
direct = RIGHT;
}
}
}
}
int main() {
init(); // 初始化地图
drawMap(); // 画出地图
while (!isGameOver()) {
control(); // 控制蛇的运动方向
move(); // 蛇的运动
drawMap(); // 画出地图
Sleep(100); // 控制蛇的速度
}
printf("Game Over!");
return 0;
}
```
这是一个基础版本的贪吃蛇游戏,您可以根据自己的需求进行修改和扩展。祝您编写愉快!
阅读全文
相关推荐
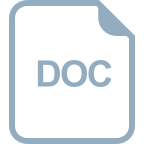
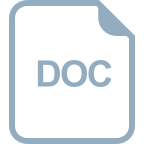
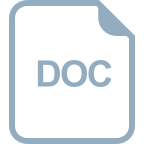
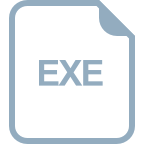




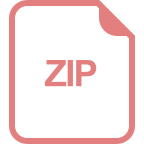




