希尔排序代码数据结构
时间: 2024-02-18 13:57:30 浏览: 61
希尔排序是一种基于插入排序的排序算法,它通过将待排序的数组分割成若干个子序列,对每个子序列进行插入排序,最后再对整个数组进行一次插入排序。希尔排序的核心思想是通过设置增量(间隔)来减少插入排序的移动次数,从而提高排序的效率。
以下是希尔排序的代码示例:
```python
def shell_sort(arr):
n = len(arr)
gap = n // 2 # 设置初始增量
while gap > 0:
for i in range(gap, n):
temp = arr[i]
j = i
while j >= gap and arr[j - gap] > temp:
arr[j] = arr[j - gap]
j -= gap
arr[j] = temp
gap //= 2 # 缩小增量
return arr
```
这段代码实现了希尔排序的交换法,通过不断缩小增量的方式,对数组进行多次插入排序,直到增量为1时完成最后一次插入排序。
相关问题
数据结构 希尔排序代码
```python
def shellSort(arr):
n = len(arr)
gap = n // 2
while gap > 0:
for i in range(gap, n):
temp = arr[i]
j = i
while j >= gap and arr[j - gap] > temp:
arr[j] = arr[j - gap]
j -= gap
arr[j] = temp
gap //= 2
arr = [12, 34, 54, 2, 3]
shellSort(arr)
print("排序后的数组:", arr) # 输出:[2, 3, 12, 34, 54]
```
c语言数据结构希尔排序代码
以下是C语言实现希尔排序的代码示例:
```c
#include <stdio.h>
#include <stdbool.h>
void shellSort(int arr[], int n) {
for (int gap = n / 2; gap > 0; gap /= 2) {
for (int i = gap; i < n; i++) {
int temp = arr[i];
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
}
}
void display(int arr[], int n) {
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
int main() {
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Input Array: ");
display(arr, n);
shellSort(arr, n);
printf("Output Array: ");
display(arr, n);
return 0;
}
```
阅读全文
相关推荐
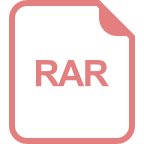
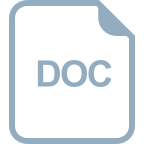
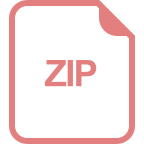
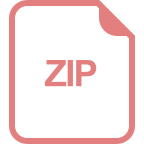
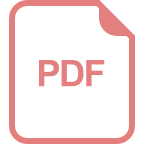
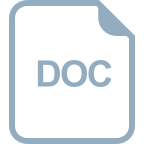
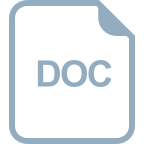
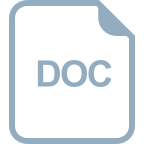
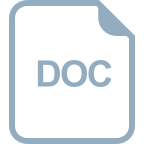



