已知平面上两点的极坐标值,且其中极角的单位是度,编写程序计算这两点之间的距离。要求所有的实数使用double类型,若需要则圆周率的近似值取3.141592653589793。 C语言
时间: 2024-10-08 22:04:35 浏览: 69
在C语言中,计算两个极坐标的点之间的距离可以利用勾股定理和极径的关系。首先,我们需要将极坐标转换成直角坐标(x, y),然后应用欧几里得距离公式。以下是计算两点间距离的一个示例函数:
```c
#include <stdio.h>
#include <math.h>
// 定义圆周率常量
#define PI 3.141592653589793
// 极坐标到笛卡尔坐标的转换函数
void polarToCartesian(double r, double theta, double* x, double* y) {
*x = r * cos(theta);
*y = r * sin(theta);
}
// 计算两点间的距离
double distance(double r1, double theta1, double r2, double theta2) {
double x1, y1, x2, y2;
// 转换极坐标为直角坐标
polarToCartesian(r1, theta1 * M_PI / 180, &x1, &y1);
polarToCartesian(r2, theta2 * M_PI / 180, &x2, &y2);
// 使用欧氏距离公式
return hypot(x2 - x1, y2 - y1);
}
int main() {
double r1, theta1, r2, theta2; // 输入的极坐标
printf("请输入第一个点的半径和极角(角度制):");
scanf("%lf %lf", &r1, &theta1);
printf("请输入第二个点的半径和极角(角度制):");
scanf("%lf %lf", &r2, &theta2);
double dist = distance(r1, theta1, r2, theta2);
printf("两点之间的距离为:%.15f\n", dist); // 输出结果保留15位小数
return 0;
}
```
阅读全文
相关推荐
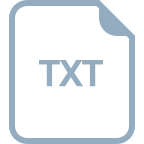
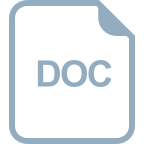




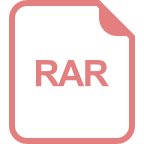
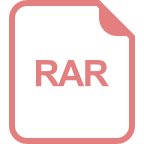
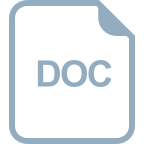

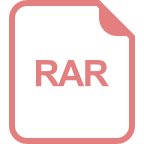
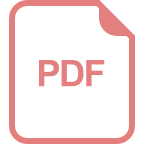
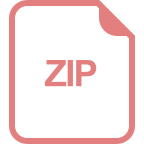
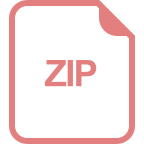