最优切割最优切割代码
时间: 2024-11-03 12:08:14 浏览: 7
最优切割问题通常是指将一块原材料分割成若干部分以获得最大价值的问题,这在很多实际场景中有应用,比如木材裁剪、玻璃切割等。它涉及到数学优化中的线性规划技术,其中一种经典的算法是动态规划。
在Python中,可以使用`scipy.optimize.linprog()`函数或` pulp`库来解决这类问题。下面是一个简单的例子:
```python
from scipy.optimize import linprog
# 假设我们有如下数据
objective = [value_of_each_piece] # 切割后的价值数组
A_ub = [[length], [width]] # 约束条件矩阵,每一行代表一条约束
b_ub = [max_size] # 上界限制
A_eq = [] # 如果有等于型约束,则在此添加
b_eq = [] # 对应的值
# 使用linprog函数
res = linprog(objective, A_ub=A_ub, b_ub=b_ub, A_eq=A_eq, b_eq=b_eq, bounds=None)
# res.x会返回每个切割部分的大小,res.fun则是总的价值
optimal_cutting_plan = res.x
max_value = res.fun
相关问题
最优切割问题代码
最优切割问题是一个经典的动态规划问题,可以使用动态规划算法来解决。下面是 Python 代码示例:
```python
def optimal_cut(n, prices):
# 创建二维数组dp,记录最优解
dp = [[0 for j in range(n)] for i in range(n)]
# 初始化dp数组
for i in range(n):
dp[i][i] = prices[i]
# 计算所有长度的最优解
for l in range(2, n + 1):
for i in range(n - l + 1):
j = i + l - 1
for k in range(i, j):
dp[i][j] = max(dp[i][j], dp[i][k] + dp[k+1][j])
dp[i][j] += prices[j] if j < n else 0
# 返回最优解
return dp[0][n-1]
```
其中,n表示原始杆的长度,prices是一个列表,表示每个位置的杆的价格。函数返回的是最优切割方案所能获得的最大总价值。
木板最优切割matlab代码
以下是一个简单的Matlab代码实现,用于解决二维矩形切割问题:
```
% 木板最优切割算法
% 输入:wood - 木板大小 [length, width]
% products - 产品信息 [length, width, num, profit]
% 输出:cuts - 木板切割方案(每块木板上的矩形) [num_wood, num_rect, x, y, w, h]
% usage - 木板使用情况 [num_wood, length, width],-1表示未使用
% profit - 总利润
function [cuts, usage, profit] = wood_cutting(wood, products)
% 计算每个产品需要的最小木板数
num_products = size(products, 1);
num_wood = size(wood, 1);
num_boards = zeros(num_products, 1);
for i = 1:num_products
num_boards(i) = ceil(products(i, 3) / max_num(wood, products(i, 1:2)));
end
% 对每个产品进行切割
cuts = cell(num_wood, num_products);
usage = -1 * ones(num_wood, wood(1), wood(2));
profit = 0;
for i = 1:num_products
for j = 1:num_boards(i)
% 在剩余木板中查找可用的矩形
max_area = 0;
max_board = 0;
max_rect = [];
for k = 1:num_wood
if usage(k, 1, 1) == -1
continue;
end
[rect, area] = find_rectangle(usage(k, :, :), products(i, 1), products(i, 2));
if area > max_area
max_area = area;
max_board = k;
max_rect = rect;
end
end
% 切割矩形
if ~isempty(max_rect)
[cuts{max_board, i}, usage(max_board, :, :)] = cut_rectangle(usage(max_board, :, :), max_rect);
profit = profit + products(i, 4);
end
end
end
end
% 计算每块木板最多可生产的产品数量
function max_num = max_num(wood, product)
max_num = floor(wood(1)/product(1)) * floor(wood(2)/product(2));
end
% 在矩阵中找到可用的矩形
function [rect, area] = find_rectangle(matrix, length, width)
[m, n] = size(matrix);
area = 0;
rect = [];
for i = 1:m-length+1
for j = 1:n-width+1
submat = matrix(i:i+length-1, j:j+width-1);
if all(submat(:) == 0)
if area == 0 || length*width < area
area = length * width;
rect = [i, j, width, length];
end
end
end
end
end
% 切割矩形
function [cuts, matrix] = cut_rectangle(matrix, rect)
[m, n] = size(matrix);
cuts = [];
for i = rect(1):rect(1)+rect(3)-1
for j = rect(2):rect(2)+rect(4)-1
cuts = [cuts; i-rect(1)+1, j-rect(2)+1, 1, 1];
matrix(i, j) = 1;
end
end
end
```
其中,函数`wood_cutting`实现了木板最优切割算法。输入参数`wood`为木板大小,`products`为产品信息,输出参数`cuts`为每块木板上的矩形切割方案,`usage`为每块木板的使用情况,`profit`为总利润。
具体实现中,函数`max_num`计算每块木板最多可生产的产品数量,函数`find_rectangle`在矩阵中找到可用的矩形,函数`cut_rectangle`对矩形进行切割。
阅读全文
相关推荐
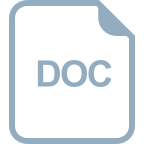
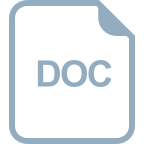
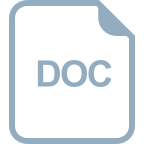



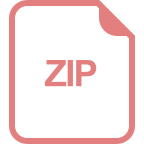
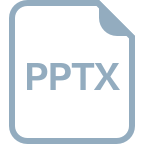
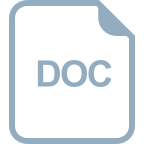






