树莓派 usb转4路485,实现串口A发文件,写一个c++demo
时间: 2024-04-14 12:26:03 浏览: 18
下面是一个使用串口A发送文件的C++示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
// 串口设备文件路径
const char* SERIAL_PORT_A = "/dev/ttyUSB0";
int main() {
// 打开串口A设备文件
int serialPortA = open(SERIAL_PORT_A, O_RDWR | O_NOCTTY);
if (serialPortA == -1) {
std::cout << "无法打开串口A设备文件" << std::endl;
return -1;
}
// 配置串口A
struct termios options;
tcgetattr(serialPortA, &options);
cfsetispeed(&options, B9600); // 设置输入波特率为9600
cfsetospeed(&options, B9600); // 设置输出波特率为9600
options.c_cflag |= (CLOCAL | CREAD); // 使能接收和本地连接
options.c_cflag &= ~PARENB; // 禁用奇偶校验
options.c_cflag &= ~CSTOPB; // 设置停止位为1
options.c_cflag &= ~CSIZE; // 清除数据位设置
options.c_cflag |= CS8; // 设置数据位为8
tcsetattr(serialPortA, TCSANOW, &options);
// 要发送的文件路径
const char* filePath = "path_to_your_file";
// 打开要发送的文件
std::ifstream file(filePath, std::ios::binary);
if (!file) {
std::cout << "无法打开文件" << filePath << std::endl;
close(serialPortA);
return -1;
}
// 发送文件内容
char buffer[1024];
while (!file.eof()) {
file.read(buffer, sizeof(buffer));
int sendBytes = write(serialPortA, buffer, file.gcount());
if (sendBytes == -1) {
std::cout << "发送文件失败" << std::endl;
break;
}
}
// 关闭文件和串口
file.close();
close(serialPortA);
return 0;
}
```
在这个示例中,我们打开了串口A设备文件并进行了配置。然后,我们打开要发送的文件,并使用循环从文件中读取数据并通过串口A发送。每次读取一定大小的数据,直到文件结束。如果发送过程中出现错误,会输出相应的提示信息。
请注意,以上代码仅提供了基本的串口发送文件的功能,并没有包括错误处理和数据传输的确认。在实际应用中,可能需要根据通信协议进行数据的处理和解析,并进行错误处理和数据传输的确认。同时,确保你已经安装了相关的串口驱动和依赖库。
相关推荐
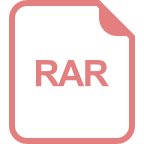
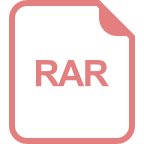














