用C++代码写一个像个平面求相交的直线方程
时间: 2024-12-17 13:36:46 浏览: 10
在C++中,计算两个平面相交的直线方程通常涉及向量运算和方程组求解。首先,我们需要定义一个结构体来存储二维空间中的直线和平面,这里以二维为例(三维类似):
```cpp
#include <iostream>
#include <vector>
struct Plane {
double a, b, c; // 定义平面 Ax + By = C 的系数
};
struct Line {
std::pair<double, double> direction; // 直线的方向向量,如(1, k)
double origin_x; // 直线的起点x坐标
};
// 计算两平面的交线方程
Line planeIntersection(const Plane& p1, const Plane& p2) {
double determinant = p1.a * p2.b - p1.b * p2.a;
if (determinant == 0) { // 平行或重合的情况
std::cout << "The planes are either parallel or coincident." << std::endl;
return Line(); // 返回空线段表示无交线
}
double intersection_x = (p1.c * p2.b - p1.b * p2.c) / determinant;
double slope = -p1.a / p1.b; // 线段的斜率
// 构造方向向量
std::pair<double, double> direction(slope, 1); // 假设我们只关心y轴方向的变化
Line result({intersection_x, direction});
return result;
}
int main() {
Plane plane1 = {1, 2, 3}; // 代表方程 x + 2y = 3
Plane plane2 = {4, 5, 6}; // 代表方程 4x + 5y = 6
Line line = planeIntersection(plane1, plane2);
if (!line.is_empty()) { // 检查是否有交线
std::cout << "The intersection line is: ";
for (const auto& coord : line.direction) {
std::cout << "(" << line.origin_x << ", " << coord.first * line.origin_x + coord.second << ")";
}
std::cout << std::endl;
}
return 0;
}
```
上述代码定义了两个平面和平面之间是否存在交线,并计算了交线的方程。注意实际应用中,可能需要处理更多边界情况和异常值。在这个例子中,`direction`实际上是单位方向向量,而真实斜率可能需要归一化。同时,`is_empty()`函数用于检查结果是否为空。
阅读全文
相关推荐
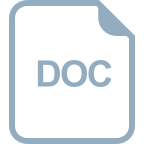
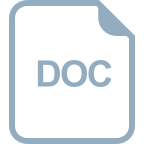
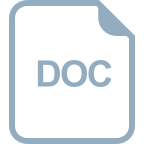

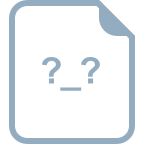
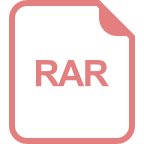

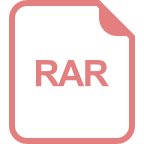
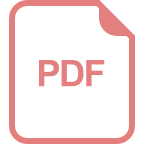
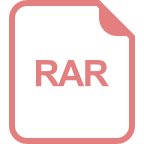
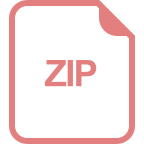
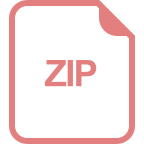
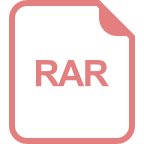
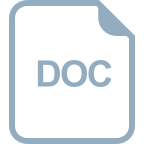
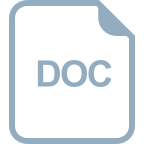
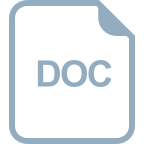
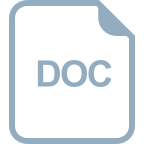


