用c语言写bmp图像的双三次插值放缩图像的代码
时间: 2024-09-10 15:07:20 浏览: 53
在C语言中实现BMP图像的双三次插值放大或缩小涉及到图像处理的一些基础知识。双三次插值是一种比较复杂的插值方法,它能够提供相对较好的放大效果,同时减少锯齿和模糊现象。以下是实现该功能的基本步骤:
1. 读取原始BMP图像的像素数据。
2. 根据目标尺寸确定插值的计算方法,通常需要对原始图像的每一个像素进行查找并计算周围16个最邻近像素的权重。
3. 计算新的像素值,这需要根据双三次插值的数学公式来进行。
4. 将计算得到的新的像素值写入到目标BMP图像中。
下面是一个简化的代码框架,用于说明如何进行双三次插值操作,但请注意,这不是一个完整的实现,而是为了展示主要步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 假设已经有了读取和保存BMP图像的函数
void LoadBMP(const char *filename, int *width, int *height, unsigned char **image);
void SaveBMP(const char *filename, int width, int height, unsigned char *image);
// 双三次插值函数
float bicubicInterpolation(float q00, float q10, float q20, float q30,
float q01, float q11, float q21, float q31,
float q02, float q12, float q22, float q32,
float q03, float q13, float q23, float q33,
float t);
int main() {
int width, height;
unsigned char *image;
// 读取原始BMP图像
LoadBMP("original.bmp", &width, &height, &image);
// 计算新的尺寸
int newWidth = width * 2; // 举例放大两倍
int newHeight = height * 2; // 举例放大两倍
// 分配内存存储新的图像数据
unsigned char *newImage = malloc(newWidth * newHeight * 3);
// 对新图像的每个像素进行双三次插值计算
for (int y = 0; y < newHeight; y++) {
for (int x = 0; x < newWidth; x++) {
// 这里需要根据x,y和新旧图像尺寸关系进行插值计算
// 这是一个复杂的计算过程,需要考虑原始图像的像素点和权重计算
// 示例:计算插值结果并赋值给新图像的像素
// newImage[(y * newWidth + x) * 3] = ...; // RGB值
}
}
// 保存新图像
SaveBMP("scaled.bmp", newWidth, newHeight, newImage);
// 释放内存资源
free(image);
free(newImage);
return 0;
}
// 这里需要实现双三次插值的计算函数bicubicInterpolation
// 该函数的实现会涉及到较为复杂的数学计算,这里不展开
//
阅读全文
相关推荐
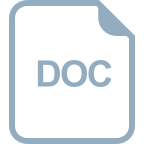
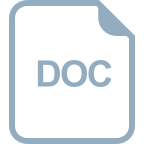
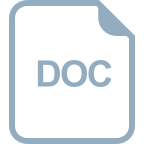

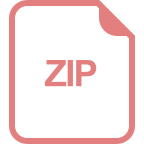
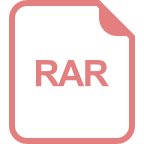



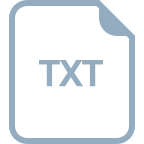
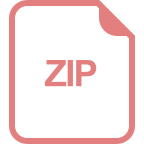
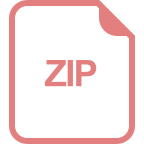



