红黑树的rotation是什么
时间: 2023-12-04 20:38:05 浏览: 37
红黑树的rotation是指通过旋转节点来保持红黑树的平衡。旋转操作有两种:左旋和右旋。左旋是指将一个节点的右子节点旋转到该节点的位置,同时该节点成为其右子节点的左子节点。右旋是指将一个节点的左子节点旋转到该节点的位置,同时该节点成为其左子节点的右子节点。旋转操作可以保持红黑树的性质不变,同时也可以使得红黑树的结构更加平衡。
相关问题
红黑树的红黑是什么意思
红黑树中的红和黑是指节点的颜色标记。在红黑树中,所有的节点都是标准的2-节点,为了体现出3-节点,红黑树使用红色链接将两个2-节点连接起来,表示一个3-节点。红色节点标记代表指向其的链接是红链接,而黑色标记的节点则是普通的节点。红黑树的定义要求从任一节点到其每个叶子的所有简单路径都包含相同数目的黑色节点。这意味着红黑树实现了一个完美的黑色平衡,如果将红黑树中所有的红色链接放平,那么所有叶子节点到根节点的距离都是相同的。因此,红黑树并不是严格的平衡二叉树,但它的综合性能非常优秀。
#### 引用[.reference_title]
- *1* *2* [清晰理解红黑树的演变---红黑的含义](https://blog.csdn.net/wy0123/article/details/79319076)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [彻底理解面试难点之rb-tree(红黑树)!!!](https://blog.csdn.net/Student_xiao_ming/article/details/87936977)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
红黑树 的红黑是指什么
红黑树的红黑指的是节点的颜色,每个节点要么是红色,要么是黑色。红黑树是一种自平衡二叉查找树,通过对节点的颜色进行限制和旋转操作来保证树的平衡性和高效性。
具体来说,红黑树要满足以下五个性质:
1. 每个节点要么是红色,要么是黑色。
2. 根节点是黑色的。
3. 每个叶子节点(NIL节点,空节点)是黑色的。
4. 如果一个节点是红色的,则它的两个子节点都是黑色的。
5. 对于每个节点,从该节点到其所有后代叶子节点的简单路径上,均包含相同数目的黑色节点。
相关推荐
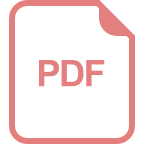
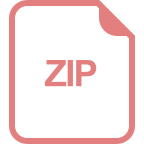
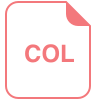
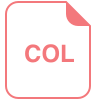
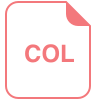
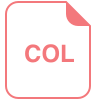
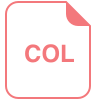







