操作系统时间片轮转调度算法 c++
时间: 2023-11-30 11:38:30 浏览: 114
时间片轮转调度算法是一种常见的进程调度算法,它将 CPU 时间分成若干个时间片,每个进程在一个时间片内运行一定时间,然后被暂停并放回就绪队列,等待下一次调度。这样可以保证每个进程都能获得一定的 CPU 时间,避免某些进程长时间占用 CPU 而导致其他进程无法运行的情况。
在实现时间片轮转调度算法时,通常需要使用一个循环队列来维护就绪队列,每次从队头取出一个进程运行,并将其放到队尾。如果一个进程的时间片用完了还没有运行完,则将其重新放到队尾,等待下一次调度。
以下是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct Process {
int pid; // 进程 ID
int burst_time; // 运行时间
int remaining_time; // 剩余时间
};
int main() {
int n, quantum; // 进程数和时间片长度
cout << "Enter the number of processes: ";
cin >> n;
cout << "Enter the time quantum: ";
cin >> quantum;
queue<Process> ready_queue; // 就绪队列
for (int i = 1; i <= n; i++) {
int burst_time;
cout << "Enter the burst time of process " << i << ": ";
cin >> burst_time;
ready_queue.push({i, burst_time, burst_time});
}
int current_time = 0; // 当前时间
while (!ready_queue.empty()) {
Process p = ready_queue.front();
ready_queue.pop();
int run_time = min(quantum, p.remaining_time); // 运行时间
current_time += run_time;
p.remaining_time -= run_time;
cout << "Process " << p.pid << " runs from " << current_time - run_time
<< " to " << current_time << endl;
if (p.remaining_time > 0) {
ready_queue.push(p);
}
}
return 0;
}
```
阅读全文
相关推荐
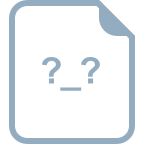
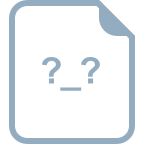
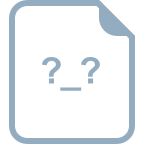
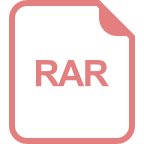









