五子棋游戏代码jupyter
时间: 2024-04-18 22:22:46 浏览: 26
五子棋是一种非常经典的棋类游戏,它的规则简单易懂,但是却有很高的策略性。下面是一个简单的五子棋游戏代码示例:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋盘状态
EMPTY = 0
BLACK = 1
WHITE = 2
class GobangGame:
def __init__(self):
self.board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
self.current_player = BLACK
def get_valid_moves(self):
valid_moves = []
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.board[i][j] == EMPTY:
valid_moves.append((i, j))
return valid_moves
def make_move(self, move):
if move in self.get_valid_moves():
self.board[move][move] = self.current_player
self.current_player = WHITE if self.current_player == BLACK else BLACK
return True
else:
return False
def check_winner(self):
directions = [(0, 1), (1, 0), (1, 1), (1, -1)]
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.board[i][j] != EMPTY:
for direction in directions:
if self.check_five_in_a_row(i, j, direction):
return self.board[i][j]
return None
def check_five_in_a_row(self, x, y, direction):
dx, dy = direction
count = 1
color = self.board[x][y]
for i in range(1, 5):
nx, ny = x + i * dx, y + i * dy
if nx < 0 or nx >= BOARD_SIZE or ny < 0 or ny >= BOARD_SIZE or self.board[nx][ny] != color:
break
count += 1
for i in range(1, 5):
nx, ny = x - i * dx, y - i * dy
if nx < 0 or nx >= BOARD_SIZE or ny < 0 or ny >= BOARD_SIZE or self.board[nx][ny] != color:
break
count += 1
return count >= 5
# 创建游戏实例
game = GobangGame()
# 游戏主循环
while True:
# 打印当前棋盘状态
print(game.board)
# 获取当前玩家的输入
x = int(input("请输入落子的横坐标:"))
y = int(input("请输入落子的纵坐标:"))
# 落子
move = (x, y)
if game.make_move(move):
# 判断是否有玩家获胜
winner = game.check_winner()
if winner is not None:
print("玩家", winner, "获胜!")
break
else:
print("无效的落子,请重新输入!")
```
这段代码使用了numpy库来表示棋盘状态,通过类`GobangGame`来管理游戏的逻辑。你可以在Jupyter Notebook中运行这段代码,然后按照提示输入横坐标和纵坐标来进行游戏。当有玩家获胜时,游戏会结束并打印获胜信息。
相关推荐
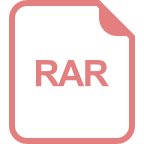
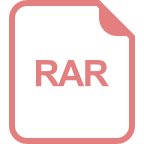














