a = tf.placeholder("float", [None, ACTIONS])TensorFlow2代码
时间: 2024-02-12 11:07:39 浏览: 22
在 TensorFlow 2.x 中,`tf.placeholder()` 已被弃用。相应的替代方法是使用 `tf.keras.Input()`。
例如,如果您想要创建一个形状为 `[None, ACTIONS]` 的输入张量,可以使用以下代码:
```
import tensorflow as tf
# 创建一个形状为 [None, ACTIONS] 的输入张量
inputs = tf.keras.Input(shape=(ACTIONS,))
# 打印张量形状
print(inputs.shape)
```
上述代码将创建一个形状为 `[None, ACTIONS]` 的输入张量,并打印其形状。请注意,因为我们将第一维设为 None,所以它可以是任意大小。如果您需要指定第一维的大小,请将其设置为一个整数值。
如果您需要在模型中使用这个张量,可以将其传递给一个 `tf.keras.layers.Layer`,例如:
```
import tensorflow as tf
# 创建一个形状为 [None, ACTIONS] 的输入张量
inputs = tf.keras.Input(shape=(ACTIONS,))
# 添加一个全连接层
x = tf.keras.layers.Dense(64, activation='relu')(inputs)
# 打印输出形状
print(x.shape)
```
上述代码将创建一个形状为 `[None, 64]` 的输出张量,这是一个全连接层的输出。
相关问题
tensorflow DRQN代码
以下是使用TensorFlow实现DRQN(Deep Recurrent Q-Network)的示例代码:
```python
import tensorflow as tf
import numpy as np
class DRQN:
def __init__(self, state_dim, action_dim, hidden_dim, batch_size, learning_rate, memory_size, max_steps):
self.state_dim = state_dim
self.action_dim = action_dim
self.hidden_dim = hidden_dim
self.batch_size = batch_size
self.learning_rate = learning_rate
self.memory_size = memory_size
self.max_steps = max_steps
self.replay_memory = []
self.build_model()
self.sess = tf.Session()
self.sess.run(tf.global_variables_initializer())
def build_model(self):
self.state_input = tf.placeholder(tf.float32, [None, self.max_steps, self.state_dim])
self.action_input = tf.placeholder(tf.int32, [None])
self.q_target = tf.placeholder(tf.float32, [None])
cell = tf.nn.rnn_cell.BasicLSTMCell(num_units=self.hidden_dim)
outputs, states = tf.nn.dynamic_rnn(cell, self.state_input, dtype=tf.float32)
output = outputs[:, -1, :]
w1 = tf.Variable(tf.random_normal([self.hidden_dim, self.action_dim]))
b1 = tf.Variable(tf.zeros([self.action_dim]))
self.q_value = tf.matmul(output, w1) + b1
self.predict_action = tf.argmax(self.q_value, axis=1)
action_one_hot = tf.one_hot(self.action_input, self.action_dim)
q_value_action = tf.reduce_sum(tf.multiply(self.q_value, action_one_hot), axis=1)
self.loss = tf.reduce_mean(tf.square(self.q_target - q_value_action))
self.optimizer = tf.train.AdamOptimizer(self.learning_rate).minimize(self.loss)
def store_experience(self, state, action, reward, next_state, done):
self.replay_memory.append((state, action, reward, next_state, done))
if len(self.replay_memory) > self.memory_size:
self.replay_memory.pop(0)
def choose_action(self, state):
if np.random.uniform() < 0.1:
return np.random.choice(self.action_dim)
else:
return self.sess.run(self.predict_action, feed_dict={self.state_input: [state]})[0]
def learn(self):
if len(self.replay_memory) < self.batch_size:
return
samples = np.random.choice(len(self.replay_memory), self.batch_size, replace=False)
states = np.zeros([self.batch_size, self.max_steps, self.state_dim])
actions = np.zeros([self.batch_size])
rewards = np.zeros([self.batch_size])
next_states = np.zeros([self.batch_size, self.max_steps, self.state_dim])
dones = np.zeros([self.batch_size])
for i, sample in enumerate(samples):
state, action, reward, next_state, done = self.replay_memory[sample]
states[i] = state
actions[i] = action
rewards[i] = reward
next_states[i] = next_state
dones[i] = done
q_values_next = self.sess.run(self.q_value, feed_dict={self.state_input: next_states})
max_q_values_next = np.max(q_values_next, axis=1)
q_targets = rewards + (1 - dones) * 0.99 * max_q_values_next
self.sess.run(self.optimizer, feed_dict={self.state_input: states, self.action_input: actions, self.q_target: q_targets})
```
这是一个简单的DRQN实现,它使用LSTM作为循环层,并且处理具有可变长度的序列输入。该模型使用经验回放进行训练,并且在每个时间步上选择动作时使用epsilon-greedy策略。
dqn代码 tensorflow
以下是一个DQN算法的TensorFlow代码示例:
```python
import tensorflow as tf
import numpy as np
import gym
# 神经网络类
class DQN:
def __init__(self, n_actions, n_features, learning_rate=0.01, reward_decay=0.9, e_greedy=0.9, replace_target_iter=300, memory_size=500, batch_size=32, e_greedy_increment=None):
self.n_actions = n_actions
self.n_features = n_features
self.lr = learning_rate
self.gamma = reward_decay
self.epsilon_max = e_greedy
self.replace_target_iter = replace_target_iter
self.memory_size = memory_size
self.batch_size = batch_size
self.epsilon_increment = e_greedy_increment
self.epsilon = 0 if e_greedy_increment is not None else self.epsilon_max
self.learn_step_counter = 0
self.memory = np.zeros((self.memory_size, n_features * 2 + 2))
self.build_net()
t_params = tf.get_collection('target_net_params')
e_params = tf.get_collection('eval_net_params')
self.replace_target_op = [tf.assign(t, e) for t, e in zip(t_params, e_params)]
self.sess = tf.Session()
self.sess.run(tf.global_variables_initializer())
self.cost_his = []
# 建立神经网络
def build_net(self):
self.s = tf.placeholder(tf.float32, [None, self.n_features], name='s')
self.q_target = tf.placeholder(tf.float32, [None, self.n_actions], name='Q_target')
with tf.variable_scope('eval_net'):
c_names = ['eval_net_params', tf.GraphKeys.GLOBAL_VARIABLES]
n_l1 = 10
w_initializer = tf.random_normal_initializer(0., 0.3)
b_initializer = tf.constant_initializer(0.1)
with tf.variable_scope('l1'):
w1 = tf.get_variable('w1', [self.n_features, n_l1], initializer=w_initializer, collections=c_names)
b1 = tf.get_variable('b1', [1, n_l1], initializer=b_initializer, collections=c_names)
l1 = tf.nn.relu(tf.matmul(self.s, w1) + b1)
with tf.variable_scope('l2'):
w2 = tf.get_variable('w2', [n_l1, self.n_actions], initializer=w_initializer, collections=c_names)
b2 = tf.get_variable('b2', [1, self.n_actions], initializer=b_initializer, collections=c_names)
self.q_eval = tf.matmul(l1, w2) + b2
with tf.variable_scope('loss'):
self.loss = tf.reduce_mean(tf.squared_difference(self.q_target, self.q_eval))
with tf.variable_scope('train'):
self._train_op = tf.train.RMSPropOptimizer(self.lr).minimize(self.loss)
self.s_ = tf.placeholder(tf.float32, [None, self.n_features], name='s_')
with tf.variable_scope('target_net'):
c_names = ['target_net_params', tf.GraphKeys.GLOBAL_VARIABLES]
n_l1 = 10
w_initializer = tf.random_normal_initializer(0., 0.3)
b_initializer = tf.constant_initializer(0.1)
with tf.variable_scope('l1'):
w1 = tf.get_variable('w1', [self.n_features, n_l1], initializer=w_initializer, collections=c_names)
b1 = tf.get_variable('b1', [1, n_l1], initializer=b_initializer, collections=c_names)
l1 = tf.nn.relu(tf.matmul(self.s_, w1) + b1)
with tf.variable_scope('l2'):
w2 = tf.get_variable('w2', [n_l1, self.n_actions], initializer=w_initializer, collections=c_names)
b2 = tf.get_variable('b2', [1, self.n_actions], initializer=b_initializer, collections=c_names)
self.q_next = tf.matmul(l1, w2) + b2
# 记忆
def store_transition(self, s, a, r, s_):
if not hasattr(self, 'memory_counter'):
self.memory_counter = 0
transition = np.hstack((s, [a, r], s_))
index = self.memory_counter % self.memory_size
self.memory[index, :] = transition
self.memory_counter += 1
# 选择动作
def choose_action(self, observation):
observation = observation[np.newaxis, :]
if np.random.uniform() < self.epsilon:
actions_value = self.sess.run(self.q_eval, feed_dict={self.s: observation})
action = np.argmax(actions_value)
else:
action = np.random.randint(0, self.n_actions)
return action
# 学习
def learn(self):
if self.learn_step_counter % self.replace_target_iter == 0:
self.sess.run(self.replace_target_op)
print('\ntarget_params_replaced\n')
if self.memory_counter > self.memory_size:
sample_index = np.random.choice(self.memory_size, size=self.batch_size)
else:
sample_index = np.random.choice(self.memory_counter, size=self.batch_size)
batch_memory = self.memory[sample_index, :]
q_next, q_eval4next = self.sess.run(
[self.q_next, self.q_eval],
feed_dict={
self.s_: batch_memory[:, -self.n_features:],
self.s: batch_memory[:, :self.n_features],
})
q_eval = self.sess.run(self.q_eval, {self.s: batch_memory[:, :self.n_features]})
q_target = q_eval.copy()
batch_index = np.arange(self.batch_size, dtype=np.int32)
eval_act_index = batch_memory[:, self.n_features].astype(int)
reward = batch_memory[:, self.n_features + 1]
q_target[batch_index, eval_act_index] = reward + self.gamma * np.max(q_next, axis=1)
_, self.cost = self.sess.run([self._train_op, self.loss],
feed_dict={self.s: batch_memory[:, :self.n_features],
self.q_target: q_target})
self.cost_his.append(self.cost)
self.epsilon = self.epsilon + self.epsilon_increment if self.epsilon < self.epsilon_max else self.epsilon_max
self.learn_step_counter += 1
# 训练网络
def main():
env = gym.make('CartPole-v0')
env = env.unwrapped
n_actions = env.action_space.n
n_features = env.observation_space.shape[0]
dqn = DQN(n_actions=n_actions, n_features=n_features, learning_rate=0.01, reward_decay=0.9, e_greedy=0.9, replace_target_iter=100, memory_size=2000, e_greedy_increment=0.001, )
for i_episode in range(400):
observation = env.reset()
ep_r = 0
while True:
env.render()
action = dqn.choose_action(observation)
observation_, reward, done, info = env.step(action)
x, x_dot, theta, theta_dot = observation_
r1 = (env.x_threshold - abs(x)) / env.x_threshold - 0.8
r2 = (env.theta_threshold_radians - abs(theta)) / env.theta_threshold_radians - 0.5
reward = r1 + r2
dqn.store_transition(observation, action, reward, observation_)
ep_r += reward
if dqn.memory_counter > dqn.memory_size:
dqn.learn()
if done:
print('episode: ', i_episode, 'ep_r: ', round(ep_r, 2), ' epsilon: ', round(dqn.epsilon, 2))
if done:
break
observation = observation_
print('game over')
env.close()
if __name__ == '__main__':
main()
```
这个代码演示了如何使用DQN算法来解决CartPole-v0游戏。在代码中,定义了一个DQN类,其中包含了神经网络的构建,记忆的存储,动作的选择和学习。在主函数中,创建了CartPole-v0环境,并使用DQN算法进行训练。
相关推荐
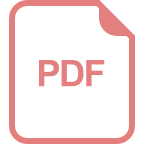
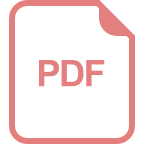












