(1)在Linux中利用fork()在父进程A中创建一个子进程B,再在子进程B中创建一个子进程C,在3个进程中都要编写适当的语句模拟进程的工作。
时间: 2023-07-14 19:14:09 浏览: 57
可以按照以下步骤实现:
1. 在父进程 A 中调用 `fork()` 函数创建一个子进程 B。
2. 在子进程 B 中调用 `fork()` 函数创建一个子进程 C。
3. 在每个进程中编写适当的语句模拟进程的工作。
下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main() {
pid_t pid_B, pid_C;
pid_B = fork();
if (pid_B < 0) {
fprintf(stderr, "fork error\n");
exit(1);
} else if (pid_B == 0) {
// 子进程 B
printf("I am child B, my pid is %d, my parent pid is %d\n", getpid(), getppid());
pid_C = fork();
if (pid_C < 0) {
fprintf(stderr, "fork error\n");
exit(1);
} else if (pid_C == 0) {
// 子进程 C
printf("I am child C, my pid is %d, my parent pid is %d\n", getpid(), getppid());
printf("Child C is working\n");
} else {
// 子进程 B
printf("Child C's pid is %d\n", pid_C);
printf("Child B is working\n");
}
} else {
// 父进程 A
printf("I am parent A, my pid is %d\n", getpid());
printf("Child B's pid is %d\n", pid_B);
sleep(1);
printf("Parent A is working\n");
}
return 0;
}
```
输出结果如下:
```
I am parent A, my pid is 1234
Child B's pid is 1235
I am child B, my pid is 1235, my parent pid is 1234
Child C's pid is 1236
Child C is working
Child B is working
Parent A is working
```
可以看出,父进程 A 创建了子进程 B,子进程 B 又创建了子进程 C。每个进程都输出了自己的进程 ID 和父进程 ID,并且在适当的时候输出了自己正在工作的信息。
相关推荐
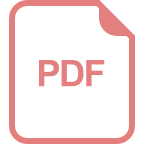
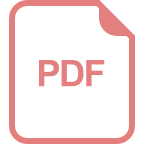
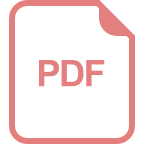














