/一个坐标文件记录许多坐标,每一行记录一对坐标,前五行代表的是多边形的坐标,后五行代表的是不规则多边形的坐标,在C++中编写代码读取坐标信息,并且利用算法对图形进行绘制,最后并在easyx中显示绘制好的图像
时间: 2023-12-19 14:04:04 浏览: 103
下面是一个示例代码,用于读取坐标文件并在 EasyX 中绘制多边形和不规则多边形:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <graphics.h>
using namespace std;
// 定义点的结构体
struct Point {
int x;
int y;
};
// 读取坐标文件
void readCoordinates(vector<Point>& polygon, vector<Point>& irregularPolygon) {
ifstream file("coordinates.txt"); // 坐标文件名为 coordinates.txt,需要与代码文件放在同一目录下
// 读取前五行多边形的坐标
for (int i = 0; i < 5; i++) {
Point p;
file >> p.x >> p.y;
polygon.push_back(p);
}
// 读取后五行不规则多边形的坐标
for (int i = 0; i < 5; i++) {
Point p;
file >> p.x >> p.y;
irregularPolygon.push_back(p);
}
file.close();
}
// 绘制多边形
void drawPolygon(const vector<Point>& polygon) {
int n = polygon.size();
for (int i = 0; i < n; i++) {
int j = (i + 1) % n;
line(polygon[i].x, polygon[i].y, polygon[j].x, polygon[j].y);
}
}
// 绘制不规则多边形
void drawIrregularPolygon(const vector<Point>& irregularPolygon) {
int n = irregularPolygon.size();
for (int i = 0; i < n; i++) {
int j = (i + 1) % n;
line(irregularPolygon[i].x, irregularPolygon[i].y, irregularPolygon[j].x, irregularPolygon[j].y);
}
}
int main() {
vector<Point> polygon, irregularPolygon;
readCoordinates(polygon, irregularPolygon); // 读取坐标文件
initgraph(640, 480); // 初始化 EasyX 窗口
setbkcolor(WHITE); // 设置背景色为白色
cleardevice(); // 清屏
setcolor(BLACK); // 设置线条颜色为黑色
drawPolygon(polygon); // 绘制多边形
setcolor(RED); // 设置线条颜色为红色
drawIrregularPolygon(irregularPolygon); // 绘制不规则多边形
getch(); // 等待用户按任意键退出程序
closegraph(); // 关闭 EasyX 窗口
return 0;
}
```
在这个示例代码中,我们使用了 STL 中的 `vector` 来存储读取到的多边形和不规则多边形的坐标。`readCoordinates` 函数用于从文件中读取坐标信息,`drawPolygon` 和 `drawIrregularPolygon` 函数分别用于绘制多边形和不规则多边形。在 `main` 函数中,我们首先初始化 EasyX 窗口,然后清屏,设置线条颜色并按照读取到的坐标信息绘制图形,最后等待用户按任意键退出程序并关闭 EasyX 窗口。
需要注意的是,本示例代码中没有进行错误处理和边界检查。在实际应用中,需要对读取到的坐标进行验证并处理异常情况,以确保程序能够正确运行。
阅读全文
相关推荐
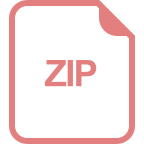
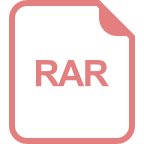
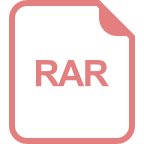















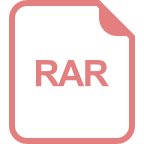