怎么对dataframe中数据分行求和
时间: 2024-01-27 15:06:35 浏览: 53
你可以使用`groupby`方法对DataFrame中的数据进行分组,并使用`sum`方法对每个组进行求和。然后,可以使用`reset_index`方法将结果重新设置为DataFrame格式。下面是一个示例代码:
```python
import pandas as pd
# 创建示例DataFrame
df = pd.DataFrame({'A': ['foo', 'bar', 'foo', 'bar', 'foo', 'bar', 'foo', 'foo'],
'B': ['one', 'one', 'two', 'two', 'two', 'one', 'one', 'two'],
'C': [1, 2, 3, 4, 5, 6, 7, 8]})
# 按列A和B进行分组,并对列C进行求和
sum_df = df.groupby(['A', 'B'])['C'].sum().reset_index()
print(sum_df)
```
这将输出按列A和B分组后的求和结果。
请注意,这是一个示例代码,你需要根据你的具体数据和需求进行修改。
相关问题
怎么对dataframe中某几行数据求和
要对DataFrame中的某几行数据进行求和,可以使用`sum`方法对指定的行进行求和。
下面是一个示例代码,假设你想要对DataFrame中的前三行进行求和:
```python
import pandas as pd
# 创建示例DataFrame
df = pd.DataFrame({'A': [1, 2, 3, 4, 5],
'B': [6, 7, 8, 9, 10],
'C': [11, 12, 13, 14, 15]})
# 对前三行数据进行求和
sum_of_rows = df.iloc[:3].sum()
print(sum_of_rows)
```
输出将是每列的求和结果。
请注意,示例中使用了`iloc`方法来选择指定的行,可以根据你的需求修改行的选择方式。
python对dataframe中数据求和、累积和、均值、标准差、方差、
Python中的pandas库提供了很多函数来对DataFrame进行统计分析,包括求和、累积和、均值、标准差、方差等。下面是一些常用的函数:
1. 求和:使用`sum()`函数,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.sum())
```
输出:
```
A 6
B 15
dtype: int64
```
2. 累积和:使用`cumsum()`函数,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.cumsum())
```
输出:
```
A B
0 1 4
1 3 9
2 6 15
```
3. 均值:使用`mean()`函数,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.mean())
```
输出:
```
A 2.0
B 5.0
dtype: float64
```
4. 标准差:使用`std()`函数,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.std())
```
输出:
```
A 1.0
B 1.0
dtype: float64
```
5. 方差:使用`var()`函数,例如:
```python
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.var())
```
输出:
```
A 1.0
B 1.0
dtype: float64
```
阅读全文
相关推荐
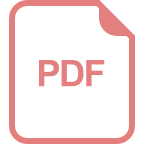
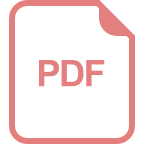
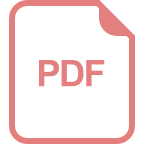
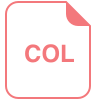
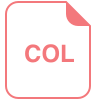











