C# Windows11API 实现蓝牙配对通信
时间: 2023-07-12 18:28:41 浏览: 259
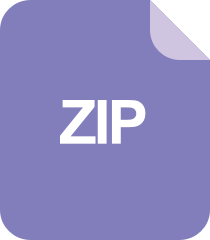
C# 蓝牙通信

要在 C# 中实现蓝牙配对通信,你需要使用 Windows 11 提供的 Bluetooth API。以下是一个简单的示例,它演示了如何在 C# 中使用 Bluetooth API 进行设备配对和通信。
首先,你需要添加对 Windows.Devices.Bluetooth 命名空间的引用。然后,你可以使用以下代码片段来开始配对过程:
```csharp
using Windows.Devices.Bluetooth;
// 获取 Bluetooth 设备
BluetoothDevice device = await BluetoothDevice.FromIdAsync(deviceId);
// 如果设备尚未配对,则开始配对过程
if (device.DeviceInformation.Pairing.CanPair)
{
// 开始配对
device.DeviceInformation.Pairing.PairAsync();
}
```
一旦设备配对成功,你就可以使用 BluetoothSocket 对象进行通信。以下是一个简单的示例,它演示了如何使用 BluetoothSocket 对象在设备之间发送数据:
```csharp
using Windows.Devices.Bluetooth.Rfcomm;
// 获取 RFCOMM 服务
RfcommDeviceService service = await RfcommDeviceService.FromIdAsync(serviceId);
// 连接到服务
StreamSocket socket = new StreamSocket();
await socket.ConnectAsync(service.ConnectionHostName, service.ConnectionServiceName);
// 发送数据
DataWriter writer = new DataWriter(socket.OutputStream);
writer.WriteString("Hello, world!");
await writer.StoreAsync();
```
这里的 serviceId 是你要连接的 RFCOMM 服务的 ID。你可以使用以下代码来获取可用的服务列表:
```csharp
using Windows.Devices.Bluetooth.GenericAttributeProfile;
// 获取 Bluetooth 设备
BluetoothLEDevice device = await BluetoothLEDevice.FromIdAsync(deviceId);
// 获取 GATT 服务列表
GattDeviceServicesResult result = await device.GetGattServicesAsync();
foreach (GattDeviceService service in result.Services)
{
// 输出服务的 UUID
Debug.WriteLine(service.Uuid.ToString());
}
```
这是一个简单的示例,它演示了如何在 C# 中使用 Windows 11 提供的 Bluetooth API 进行设备配对和通信。你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
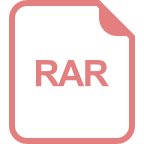
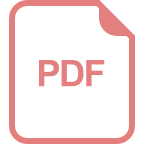








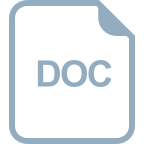
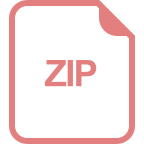
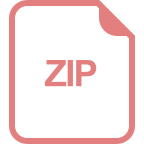
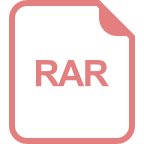
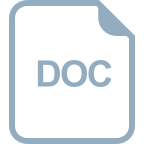
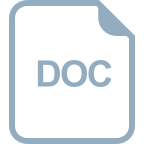
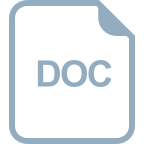
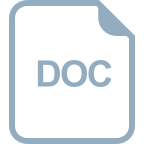