module fifo ( input clk, rst, wr_en, rd_en, input [7:0] wr_data, output [7:0] rd_data, output empty, full, almost_full, almost_empty ); parameter BUF_DEPTH = 4; reg [7:0] buffer [0:BUF_DEPTH-1]; reg [1:0] head, tail; reg [2:0] count; assign empty = (count == 0); assign full = (count == BUF_DEPTH); assign almost_full = (count == BUF_DEPTH-1); assign almost_empty = (count == 1); always @(posedge clk or negedge rst) begin if(!rst) begin head <= 0; tail <= 0; count <= 0; end else begin if(wr_en && !full) begin buffer[head] <= wr_data; head <= (head + 1) % BUF_DEPTH; count <= count + 1; end if(rd_en && !empty) begin rd_data <= buffer[tail]; tail <= (tail + 1) % BUF_DEPTH; count <= count - 1; end end end endmodule
时间: 2024-04-26 13:23:55 浏览: 81
这段代码是一个Verilog实现的FIFO模块,它的作用是实现一个先进先出的数据缓存。模块的输入输出和标志如下:
输入:
- clk:时钟信号;
- rst:复位信号;
- wr_en:写入使能信号;
- rd_en:读出使能信号;
- wr_data:写入的数据。
输出:
- rd_data:读出的数据;
- empty:FIFO为空标志;
- full:FIFO为满标志;
- almost_full:FIFO几乎为满标志;
- almost_empty:FIFO几乎为空标志。
参数:
- BUF_DEPTH:FIFO的深度。
具体实现:
- buffer:用于存储队列数据的数组;
- head:指向队列头部的索引;
- tail:指向队列尾部的索引;
- count:队列中元素的数量。
FIFO模块的实现采用了时序逻辑,使用了always块,对于时钟上升沿的变化和复位信号的变化作出反应。在时钟上升沿时,如果写使能为1且队列未满,将数据写入队列中;如果读使能为1且队列不为空,从队列中读取数据。如果复位信号为0,则将队列清空。
阅读全文
相关推荐


















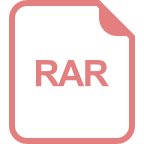
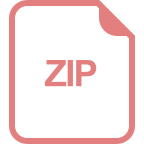