写一个五子棋的人机博弈python程序
时间: 2023-09-15 11:20:39 浏览: 59
好的,我可以给您提供一个基本的五子棋人机博弈Python程序。以下是程序代码:
```python
import numpy as np
import random
# 定义棋盘大小和赢法数组
BOARD_SIZE = 15
WIN_COUNT = 5
wins = np.zeros((BOARD_SIZE, BOARD_SIZE, 572), dtype=int)
# 初始化赢法数组
def init_wins():
count = 0
# 横向赢法
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - WIN_COUNT + 1):
for k in range(WIN_COUNT):
wins[i][j+k][count] = 1
count += 1
# 纵向赢法
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - WIN_COUNT + 1):
for k in range(WIN_COUNT):
wins[j+k][i][count] = 1
count += 1
# 斜向赢法
for i in range(BOARD_SIZE - WIN_COUNT + 1):
for j in range(BOARD_SIZE - WIN_COUNT + 1):
for k in range(WIN_COUNT):
wins[i+k][j+k][count] = 1
count += 1
# 反斜向赢法
for i in range(WIN_COUNT - 1, BOARD_SIZE):
for j in range(BOARD_SIZE - WIN_COUNT + 1):
for k in range(WIN_COUNT):
wins[i-k][j+k][count] = 1
count += 1
return count
# 初始化棋盘
def init_board():
board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
return board
# 人下棋
def human_play(board, x, y):
if board[x][y] == 0:
board[x][y] = 1
return True
else:
return False
# AI下棋
def ai_play(board):
empty_points = np.argwhere(board == 0)
scores = []
for point in empty_points:
score = 0
for i in range(len(wins[point[0]][point[1]])):
if wins[point[0]][point[1]][i] == 1:
score += 1
scores.append(score)
max_score = max(scores)
max_points = [empty_points[i] for i in range(len(scores)) if scores[i] == max_score]
ai_point = random.choice(max_points)
board[ai_point[0]][ai_point[1]] = 2
return ai_point[0], ai_point[1]
# 判断是否结束
def is_gameover(board, x, y):
for i in range(len(wins[x][y])):
if wins[x][y][i] == 1:
count = 0
for j in range(WIN_COUNT):
if wins[x][y+j-WIN_COUNT+1][i] == 1 and board[x][y+j-WIN_COUNT+1] == board[x][y]:
count += 1
if count == WIN_COUNT:
return True
count = 0
for j in range(WIN_COUNT):
if wins[x+j-WIN_COUNT+1][y][i] == 1 and board[x+j-WIN_COUNT+1][y] == board[x][y]:
count += 1
if count == WIN_COUNT:
return True
count = 0
for j in range(WIN_COUNT):
if wins[x+j-WIN_COUNT+1][y+j-WIN_COUNT+1][i] == 1 and board[x+j-WIN_COUNT+1][y+j-WIN_COUNT+1] == board[x][y]:
count += 1
if count == WIN_COUNT:
return True
count = 0
for j in range(WIN_COUNT):
if wins[x+j-WIN_COUNT+1][y+j-WIN_COUNT+1+WIN_COUNT-1-2*(j-WIN_COUNT+1)][i] == 1 and board[x+j-WIN_COUNT+1][y+j-WIN_COUNT+1+WIN_COUNT-1-2*(j-WIN_COUNT+1)] == board[x][y]:
count += 1
if count == WIN_COUNT:
return True
return False
# 打印棋盘
def print_board(board):
print(" ", end="")
for i in range(BOARD_SIZE):
print(i, end=" ")
print("")
for i in range(BOARD_SIZE):
print(i, end=" ")
for j in range(BOARD_SIZE):
if board[i][j] == 0:
print("+", end=" ")
elif board[i][j] == 1:
print("O", end=" ")
elif board[i][j] == 2:
print("X", end=" ")
print("")
# 主函数
def main():
count = init_wins()
board = init_board()
print_board(board)
while True:
x = int(input("请输入您下棋的横坐标:"))
y = int(input("请输入您下棋的纵坐标:"))
if human_play(board, x, y):
if is_gameover(board, x, y):
print_board(board)
print("您赢了!")
break
ai_x, ai_y = ai_play(board)
print("AI下棋:(%d, %d)" % (ai_x, ai_y))
print_board(board)
if is_gameover(board, ai_x, ai_y):
print("AI赢了!")
break
else:
print("此处已有棋子,请重新输入。")
if __name__ == '__main__':
main()
```
这个程序实现了基本的五子棋人机博弈,您可以在此基础上进行修改和完善。
相关推荐
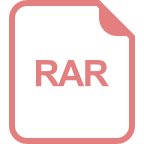
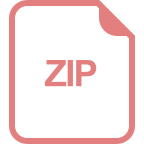
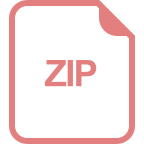
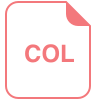













