用c语言编程 输入年、月、日,输出该日期是当年的第几天,考虑闰年和平年情况。 输入格式: 三个整数,分别表示年、月、日,以空格分隔。 输出格式: 一个整数,表示输入日期是当年的第几天。
时间: 2024-11-20 16:40:07 浏览: 17
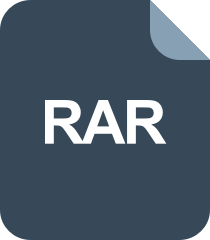
输入日期计算这是一年中第几天C语言源代码.rar
在C语言中,你可以编写一个函数来计算给定日期是一年的第几天,考虑到闰年和平年的规则。首先,我们需要定义闰年的条件:能被4整除但不能被100整除的年份是闰年,除非它也能被400整除。然后按照每个月的天数来累加。以下是关键步骤:
1. 定义一个判断是否为闰年的函数,如`is_leap_year(int year)`。
2. 定义一个函数`days_in_month(int month, int year)`,返回指定月份的天数。
3. 主函数`main()`中获取用户输入的年、月、日,计算总天数。
下面是一个简单的示例代码:
```c
#include <stdio.h>
// 判断是否为闰年
int is_leap_year(int year) {
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
return 1;
}
return 0;
}
// 按照月份计算天数
int days_in_month(int month, int year) {
switch (month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2:
// 考虑闰年
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default:
return -1; // 错误的月份
}
}
// 计算特定日期是一年中的第几天
int day_of_year(int year, int month, int day) {
int days = 0;
for (int i = 1; i < month; i++) {
days += days_in_month(i, year);
}
days += day;
// 如果是闰年且二月需要额外一天
if (is_leap_year(year) && month > 2) {
days++;
}
return days;
}
int main() {
int year, month, day;
printf("请输入年月日(空格分隔):");
scanf("%d %d %d", &year, &month, &day);
if (month < 1 || month > 12 || day < 1 || day > days_in_month(month, year)) {
printf("错误的日期输入!\n");
} else {
int result = day_of_year(year, month, day);
printf("输入日期是当年的第%d天。\n", result);
}
return 0;
}
```
阅读全文
相关推荐
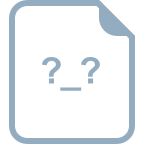
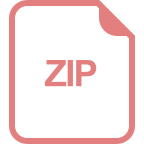















