已知三维上的两点坐标,确定一条直线,输入x,y,首先判断x,y是否在直线水平面投影上,若在求z,根据上述要求,写一段C++代码,要求将点封装为结构体
时间: 2024-05-03 07:21:27 浏览: 4
以下是C++代码(结构体Point包含三个成员变量x、y、z):
```cpp
#include <iostream>
#include <cmath>
using namespace std;
struct Point {
double x;
double y;
double z;
};
struct Line {
Point p1;
Point p2;
};
bool isOnHorizontal(Line line, Point point) {
double x1 = line.p1.x, y1 = line.p1.y, x2 = line.p2.x, y2 = line.p2.y;
double px = point.x, py = point.y;
double k = (y2 - y1) / (x2 - x1);
double b = y1 - k * x1;
double h = k * px + b;
return fabs(h - py) < 1e-6;
}
bool isOnLine(Line line, Point point) {
double x1 = line.p1.x, y1 = line.p1.y, z1 = line.p1.z;
double x2 = line.p2.x, y2 = line.p2.y, z2 = line.p2.z;
double px = point.x, py = point.y;
double k = (z2 - z1) / sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2));
double b = z1 - k * sqrt(pow(px - x1, 2) + pow(py - y1, 2));
point.z = k * sqrt(pow(px - x1, 2) + pow(py - y1, 2)) + b;
return isOnHorizontal(line, point);
}
int main() {
Point point = {2, 3, 0};
Line line = {{1, 1, 1}, {4, 4, 4}};
if (isOnLine(line, point)) {
cout << "The point is on the line." << endl;
cout << "z = " << point.z << endl;
} else {
cout << "The point is not on the line." << endl;
}
return 0;
}
```
首先定义了两个结构体Point和Line,分别表示点和线。isOnHorizontal函数判断点是否在直线的水平面投影上,isOnLine函数根据点的x、y坐标计算出z坐标,并判断点是否在直线上。在main函数中测试了一个点是否在指定的直线上,如果在则输出z坐标,否则输出不在直线上的信息。
相关推荐
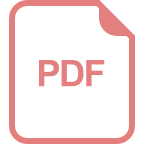














