ffmpeg打开输入流缓冲区
时间: 2023-10-11 09:12:12 浏览: 52
FFmpeg通过使用avformat_open_input()函数来打开输入媒体流并读取头部信息,包括本地文件、网络流和自定义缓冲区。这个函数会打开avio,然后探测输入流的封装格式。具体地说,avformat_open_input()函数会调用avio_alloc_context()函数来分配AVIOContext,其中包括分配缓冲区buffer。这个缓冲区用于读取和写入媒体数据。所以,当使用ffmpeg打开输入流时,会分配一个缓冲区来处理数据。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关问题
FFmpeg推流命令
FFmpeg是一个开源的跨平台音视频处理工具,可以用来进行音视频的编解码、转码、剪辑、推流等操作。下面是一个简单的推流命令示例:
```
ffmpeg -re -i input.mp4 -c:v libx264 -preset veryfast -b:v 3000k -maxrate 3000k -bufsize 6000k -c:a aac -b:a 128k -f flv rtmp://server/live/stream
```
其中,`-re` 表示以实时模式推流,`-i input.mp4` 表示输入文件为 `input.mp4`,`-c:v libx264` 表示使用 H.264 编码器进行视频编码,`-preset veryfast` 表示编码速度优先,`-b:v 3000k` 表示视频码率为 3000kbps,`-maxrate 3000k` 表示最大视频码率为 3000kbps,`-bufsize 6000k` 表示视频缓冲区大小为 6000kb,`-c:a aac` 表示使用 AAC 编码器进行音频编码,`-b:a 128k` 表示音频码率为 128kbps,`-f flv` 表示输出格式为 FLV,`rtmp://server/live/stream` 表示推流地址为 `rtmp://server/live/stream`。
ffmpeg 推拉流 代码
FFmpeg是一个开源的跨平台音视频处理工具,可以用于音视频的编解码、转码、推拉流等操作。下面是一个简单的示例代码,用于推送和拉取流:
1. 推流代码示例:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
int main(int argc, char *argv[]) {
AVFormatContext *fmt_ctx = NULL;
AVOutputFormat *out_fmt = NULL;
AVStream *video_stream = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVFrame *frame = NULL;
AVPacket pkt;
const char *output_url = "rtmp://example.com/live/stream";
av_register_all();
// 初始化输出格式上下文
avformat_alloc_output_context2(&fmt_ctx, NULL, "flv", output_url);
if (!fmt_ctx) {
printf("Failed to allocate output context\n");
return -1;
}
out_fmt = fmt_ctx->oformat;
// 添加视频流
video_stream = avformat_new_stream(fmt_ctx, NULL);
if (!video_stream) {
printf("Failed to create video stream\n");
return -1;
}
codec_ctx = video_stream->codec;
// 设置编码器参数
codec_ctx->codec_id = out_fmt->video_codec;
codec_ctx->codec_type = AVMEDIA_TYPE_VIDEO;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
codec_ctx->width = 640;
codec_ctx->height = 480;
codec_ctx->bit_rate = 400000;
codec_ctx->gop_size = 10;
// 初始化编码器
codec = avcodec_find_encoder(codec_ctx->codec_id);
if (!codec) {
printf("Failed to find encoder\n");
return -1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
printf("Failed to open encoder\n");
return -1;
}
// 打开输出URL
if (avio_open(&fmt_ctx->pb, output_url, AVIO_FLAG_WRITE) < 0) {
printf("Failed to open output URL\n");
return -1;
}
// 写入文件头
if (avformat_write_header(fmt_ctx, NULL) < 0) {
printf("Failed to write header\n");
return -1;
}
// 初始化帧
frame = av_frame_alloc();
frame->format = codec_ctx->pix_fmt;
frame->width = codec_ctx->width;
frame->height = codec_ctx->height;
// 分配帧数据缓冲区
int ret = av_frame_get_buffer(frame, 0);
if (ret < 0) {
printf("Failed to allocate frame buffer\n");
return -1;
}
// 推送视频帧
for (int i = 0; i < 100; i++) {
// 生成测试图像数据
// ...
// 将图像数据填充到帧中
// ...
// 编码帧
// ...
// 将编码后的数据写入输出流
av_interleaved_write_frame(fmt_ctx, &pkt);
av_packet_unref(&pkt);
}
// 写入文件尾
av_write_trailer(fmt_ctx);
// 清理资源
av_frame_free(&frame);
avcodec_close(codec_ctx);
avio_close(fmt_ctx->pb);
avformat_free_context(fmt_ctx);
return 0;
}
```
2. 拉流代码示例:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
int main(int argc, char *argv[]) {
AVFormatContext *fmt_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVPacket pkt;
AVFrame *frame = NULL;
const char *input_url = "rtmp://example.com/live/stream";
av_register_all();
// 打开输入URL
if (avformat_open_input(&fmt_ctx, input_url, NULL, NULL) != 0) {
printf("Failed to open input URL\n");
return -1;
}
// 获取流信息
if (avformat_find_stream_info(fmt_ctx, NULL) < 0) {
printf("Failed to find stream info\n");
return -1;
}
// 查找视频流索引
int video_stream_index = -1;
for (int i = 0; i < fmt_ctx->nb_streams; i++) {
if (fmt_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
printf("Failed to find video stream\n");
return -1;
}
// 获取视频流解码器参数
codec_ctx = avcodec_alloc_context3(NULL);
if (!codec_ctx) {
printf("Failed to allocate codec context\n");
return -1;
}
if (avcodec_parameters_to_context(codec_ctx, fmt_ctx->streams[video_stream_index]->codecpar) < 0) {
printf("Failed to copy codec parameters to context\n");
return -1;
}
// 查找解码器
codec = avcodec_find_decoder(codec_ctx->codec_id);
if (!codec) {
printf("Failed to find decoder\n");
return -1;
}
// 打开解码器
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
printf("Failed to open decoder\n");
return -1;
}
// 初始化帧
frame = av_frame_alloc();
// 读取视频帧
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == video_stream_index) {
// 解码帧
// ...
// 处理解码后的数据
// ...
}
av_packet_unref(&pkt);
}
// 清理资源
av_frame_free(&frame);
avcodec_close(codec_ctx);
avformat_close_input(&fmt_ctx);
return 0;
}
```
这是一个简单的示例代码,用于推送和拉取流。实际使用时,还需要根据具体需求进行参数配置和错误处理。
相关推荐
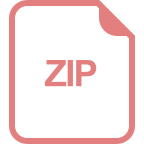
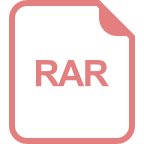












