数据结构中使用C++语言编程一个关于实现线性表的抽象数据类型的代码
时间: 2024-10-04 10:01:44 浏览: 39
在C++中,为了实现一个线性表的抽象数据类型,通常我们会创建一个模板类,比如双向链表或数组形式的线性表。这里以单向链表为例,展示如何实现基本操作如插入、删除和访问节点:
```cpp
template <typename T>
class LinkedList {
private:
struct Node {
T data;
Node* next;
Node(T value = T()) : data(value), next(nullptr) {}
};
public:
LinkedList() : head(nullptr) {}
// 插入元素到链表头部
void pushFront(const T& value) {
Node* newNode = new Node(value);
newNode->next = head;
head = newNode;
}
// 插入元素到链表尾部
void pushBack(const T& value) {
if (head == nullptr) {
head = new Node(value);
return;
}
Node* current = head;
while (current->next != nullptr)
current = current->next;
current->next = new Node(value);
}
// 删除指定值的第一个匹配项
void remove(const T& value) {
if (head == nullptr || head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next != nullptr && current->next->data != value)
current = current->next;
if (current->next != nullptr)
current->next = current->next->next;
else
cout << "Value not found!" << endl;
}
// 访问特定索引的元素
T& operator[](size_t index) {
if (index >= size())
throw std::out_of_range("Index out of range");
Node* current = head;
for (size_t i = 0; i < index; ++i)
current = current->next;
return current->data;
}
// 返回链表大小
size_t size() const {
size_t count = 0;
Node* current = head;
while (current != nullptr) {
++count;
current = current->next;
}
return count;
}
private:
Node* head;
};
```
阅读全文
相关推荐
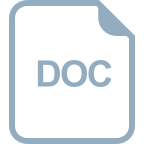
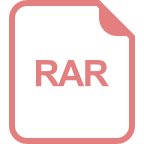
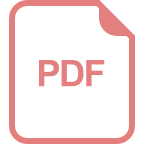
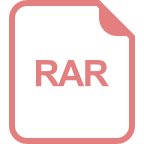
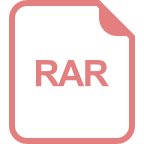
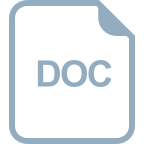
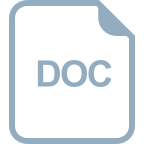
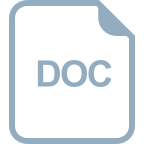
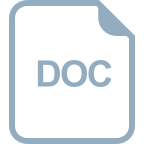
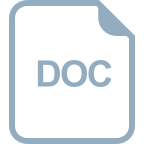
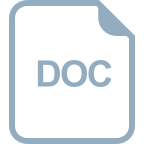
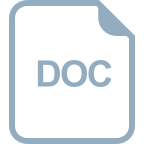
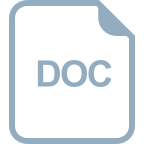
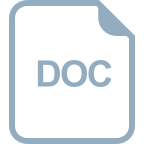
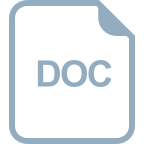
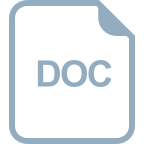
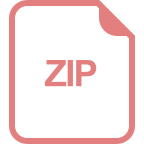
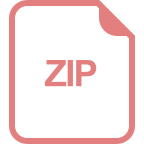