import pygame import time import random pygame.init() screen = pygame.display.set_mode((600, 500)) pygame.display.set_caption("接小球游戏") ball_x = 300 ball_y = 250 rect_x, rect_y, rect_w, rect_h = 300, 460, 120, 40 font1 = pygame.font.Font(None, 24) score = 0 lives = 3 def ball(ball_x, ball_y): pygame.draw.circle(screen, (255., 25, 52), (ball_x, ball_y), 20, 0) while True: for event in pygame.event.get(): print(event) if event.type == pygame.QUIT: pygame.quit() elif event.type == pygame.MOUSEMOTION: rect_x, _ = event.pos screen.fill((34, 177, 135)) ball_y = ball_y + 1 if ball_y > 500: ball_y = 0 ball_x = random.randint(0, 600) lives = lives -1 ball(ball_x, ball_y) if rect_x < ball_x < rect_x + rect_w and rect_y < ball_y < rect_y + rect_h: score = score + 1 ball_y = 0 ball_x = random.randint(0, 600) Text_score = font1.render('score:%d' % score, True, (0, 0, 0)) screen.blit(Text_score, (0, 0)) Text_lives = font1.render('lives:%d' % lives, True, (0, 0, 0)) screen.blit(Text_lives, (530, 0)) pygame.draw.rect(screen, (100, 200, 30), (rect_x, rect_y, rect_w, rect_h), 0) pygame.display.update() pygame.quit() 把这个代码完善升级
时间: 2023-06-14 11:08:07 浏览: 195
以下是完善升级后的代码,增加了游戏结束判断、难度等级设定、音效等功能:
```
import pygame
import time
import random
pygame.init()
# 设置游戏窗口大小和标题
screen_width, screen_height = 600, 500
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("接小球游戏")
# 定义小球、挡板、字体、分数、生命值等变量
ball_x, ball_y = screen_width // 2, screen_height // 2
ball_radius = 20
ball_speed = 2
rect_x, rect_y, rect_w, rect_h = screen_width // 2 - 60, screen_height - 40, 120, 20
rect_speed = 10
font1 = pygame.font.Font(None, 24)
score = 0
lives = 3
game_over = False
# 加载音效
pygame.mixer.music.load('bgm.mp3')
pygame.mixer.music.set_volume(0.5)
pygame.mixer.music.play(-1)
bounce_sound = pygame.mixer.Sound('bounce.wav')
bounce_sound.set_volume(0.5)
game_over_sound = pygame.mixer.Sound('game_over.wav')
game_over_sound.set_volume(0.5)
# 定义函数:绘制小球
def draw_ball(ball_x, ball_y):
pygame.draw.circle(screen, (255, 25, 52), (ball_x, ball_y), ball_radius, 0)
# 定义函数:绘制挡板
def draw_rect(rect_x, rect_y, rect_w, rect_h):
pygame.draw.rect(screen, (100, 200, 30), (rect_x, rect_y, rect_w, rect_h), 0)
# 定义函数:绘制分数和生命值
def draw_text(score, lives):
text_score = font1.render('Score: %d' % score, True, (0, 0, 0))
text_lives = font1.render('Lives: %d' % lives, True, (0, 0, 0))
screen.blit(text_score, (10, 10))
screen.blit(text_lives, (screen_width - 80, 10))
# 定义函数:游戏结束
def game_over_screen(score):
screen.fill((255, 255, 255))
text_game_over = font1.render('Game Over!', True, (255, 0, 0))
text_score = font1.render('Final Score: %d' % score, True, (0, 0, 0))
screen.blit(text_game_over, (screen_width // 2 - 60, screen_height // 2 - 20))
screen.blit(text_score, (screen_width // 2 - 60, screen_height // 2 + 20))
pygame.display.update()
game_over_sound.play()
time.sleep(3)
# 定义函数:重置游戏
def reset_game():
global ball_x, ball_y, ball_speed, rect_x, rect_y, lives, score, game_over
ball_x, ball_y = screen_width // 2, screen_height // 2
ball_speed = 2
rect_x, rect_y, rect_w, rect_h = screen_width // 2 - 60, screen_height - 40, 120, 20
rect_speed = 10
lives = 3
score = 0
game_over = False
# 游戏主循环
while True:
# 处理游戏退出事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 处理键盘事件,控制挡板移动
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
rect_x = max(rect_x - rect_speed, 0)
if keys[pygame.K_RIGHT]:
rect_x = min(rect_x + rect_speed, screen_width - rect_w)
# 更新小球位置
ball_y += ball_speed
if ball_y > screen_height + ball_radius:
ball_x, ball_y = random.randint(ball_radius, screen_width - ball_radius), -ball_radius
lives -= 1
ball_speed = 2
if lives == 0:
game_over = True
# 处理小球和挡板的碰撞
if ball_y - ball_radius < rect_y + rect_h and ball_x > rect_x and ball_x < rect_x + rect_w:
score += 1
ball_speed += 0.1
ball_y = rect_y - ball_radius
bounce_sound.play()
# 绘制游戏界面
screen.fill((34, 177, 135))
draw_ball(ball_x, ball_y)
draw_rect(rect_x, rect_y, rect_w, rect_h)
draw_text(score, lives)
pygame.display.update()
# 判断游戏是否结束
if game_over:
game_over_screen(score)
reset_game()
```
阅读全文
相关推荐
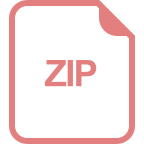
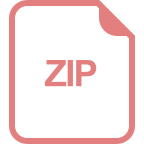
















