如何一次读取多个Excel文件并合并
时间: 2023-07-28 11:06:20 浏览: 59
可以使用Python的pandas库来一次读取多个Excel文件并合并。具体步骤如下:
1. 导入pandas库和os库
```python
import pandas as pd
import os
```
2. 设置文件夹路径和文件后缀名
```python
folder_path = 'path/to/folder'
file_extension = '.xlsx'
```
3. 遍历文件夹,找到所有符合后缀名的文件
```python
file_list = [file for file in os.listdir(folder_path) if file.endswith(file_extension)]
```
4. 循环读取每个Excel文件,并将它们合并成一个DataFrame
```python
df_list = []
for file in file_list:
df = pd.read_excel(os.path.join(folder_path, file))
df_list.append(df)
merged_df = pd.concat(df_list, ignore_index=True)
```
5. 可以根据需要对合并后的DataFrame进行进一步处理,如保存为新的Excel文件
```python
merged_df.to_excel('path/to/output_file.xlsx', index=False)
```
完整代码示例:
```python
import pandas as pd
import os
folder_path = 'path/to/folder'
file_extension = '.xlsx'
file_list = [file for file in os.listdir(folder_path) if file.endswith(file_extension)]
df_list = []
for file in file_list:
df = pd.read_excel(os.path.join(folder_path, file))
df_list.append(df)
merged_df = pd.concat(df_list, ignore_index=True)
merged_df.to_excel('path/to/output_file.xlsx', index=False)
```
相关推荐
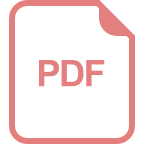
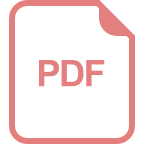
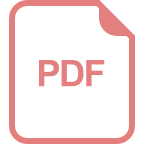













