C语言读写陀螺仪姿态算法代码
时间: 2023-09-10 12:07:20 浏览: 62
陀螺仪姿态算法一般需要涉及到数学计算和向量运算,因此需要依赖一些数学库和向量库。以下是一个读取陀螺仪数据并实现姿态算法的示例代码:
```
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
typedef struct {
double x, y, z;
} Vector3D;
Vector3D crossProduct(Vector3D a, Vector3D b) {
Vector3D result;
result.x = a.y * b.z - a.z * b.y;
result.y = a.z * b.x - a.x * b.z;
result.z = a.x * b.y - a.y * b.x;
return result;
}
double dotProduct(Vector3D a, Vector3D b) {
return a.x * b.x + a.y * b.y + a.z * b.z;
}
double magnitude(Vector3D v) {
return sqrt(v.x * v.x + v.y * v.y + v.z * v.z);
}
Vector3D normalize(Vector3D v) {
double mag = magnitude(v);
Vector3D result;
result.x = v.x / mag;
result.y = v.y / mag;
result.z = v.z / mag;
return result;
}
void updateOrientation(Vector3D angularVelocity, double timeStep, Vector3D* orientation) {
Vector3D deltaOrientation = crossProduct(*orientation, angularVelocity);
deltaOrientation.x *= timeStep;
deltaOrientation.y *= timeStep;
deltaOrientation.z *= timeStep;
*orientation = normalize((Vector3D) {
orientation->x + deltaOrientation.x / 2.0,
orientation->y + deltaOrientation.y / 2.0,
orientation->z + deltaOrientation.z / 2.0
});
}
int main() {
Vector3D angularVelocity = {0.1, 0.2, 0.3};
double timeStep = 0.01;
Vector3D orientation = {1.0, 0.0, 0.0};
for (int i = 0; i < 1000; i++) {
updateOrientation(angularVelocity, timeStep, &orientation);
printf("Orientation: (%f, %f, %f)\n", orientation.x, orientation.y, orientation.z);
}
return 0;
}
```
这个示例代码中,我们定义了一个 `Vector3D` 结构体来表示三维向量,然后实现了一些向量运算的函数,包括叉乘、点乘、求模长和归一化。在 `updateOrientation` 函数中,我们根据陀螺仪数据计算出角速度,并根据时间步长计算出姿态变化,最后更新姿态。在 `main` 函数中,我们简单地模拟了陀螺仪数据,并多次调用 `updateOrientation` 函数来更新姿态。
相关推荐
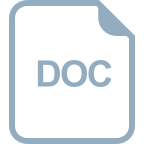














