如何将xlsx文件中的json字段转换为xlsx格式
时间: 2024-11-30 21:13:42 浏览: 21
将Excel (.xlsx) 文件中的 JSON 数据转换回 Excel 格式通常涉及到两个步骤:首先,你需要读取包含 JSON 字段的 Excel 文件并解析其内容,然后将 JSON 数据转换成适合的 Excel 表格结构,并保存为新的 Excel 文件。
1. **读取Excel并提取JSON数据**:
- 使用Python的`pandas`库可以方便地读取`.xlsx`文件,如 `pd.read_excel('input.xlsx')`。
- 对于包含JSON数据的特定单元格,可能需要额外处理,例如使用`ast.literal_eval()`函数解析字符串化的JSON。
```python
import pandas as pd
import json
# 读取Excel文件
df = pd.read_excel('input.xlsx')
# 假设有一个名为 'json_column' 的列包含JSON数据
data_json_column = df['json_column']
```
2. **解析JSON并创建DataFrame**:
- 将JSON数据转换成Python字典列表,然后构建一个新的`pandas.DataFrame`。
```python
# 解析每个JSON字符串为字典
json_dicts = [json.loads(row) for row in data_json_column]
# 构建新的DataFrame
new_df = pd.json_normalize(json_dicts)
```
3. **合并原始数据与JSON数据**:
- 如果需要,你可以将新 DataFrame 合并到原始 DataFrame 中。
```python
df_with_json = pd.concat([df.drop('json_column', axis=1), new_df], axis=1)
```
4. **保存为新的Excel文件**:
- 最后,使用`to_excel()`方法将结果保存为`.xlsx`格式。
```python
df_with_json.to_excel('output.xlsx', index=False)
```
阅读全文
相关推荐
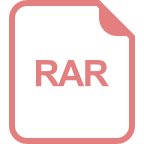
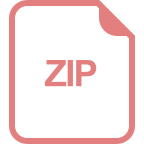
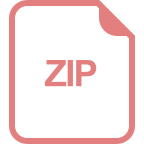
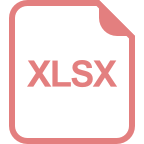
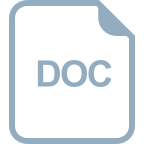
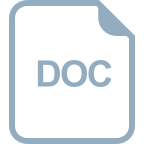
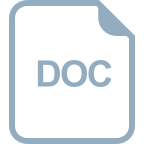
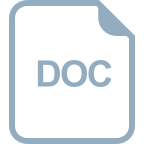











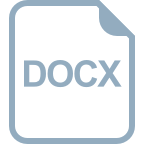