定义一个简单形体(OpenGL提供的基础形体,如立方体、球体等),并生成正交投影及透视投影视图
时间: 2023-11-22 16:55:54 浏览: 178
以下是使用OpenGL生成立方体并生成正交投影和透视投影视图的示例代码:
```c++
#include <GL/glut.h>
// 定义立方体顶点坐标
GLfloat vertices[] = {
-1.0f, -1.0f, -1.0f,
1.0f, -1.0f, -1.0f,
1.0f, 1.0f, -1.0f,
-1.0f, 1.0f, -1.0f,
-1.0f, -1.0f, 1.0f,
1.0f, -1.0f, 1.0f,
1.0f, 1.0f, 1.0f,
-1.0f, 1.0f, 1.0f
};
// 定义立方体面索引
GLubyte indices[] = {
0, 1, 2, 3,
3, 2, 6, 7,
7, 6, 5, 4,
4, 5, 1, 0,
0, 3, 7, 4,
1, 2, 6, 5
};
// 定义正交投影和透视投影的视角参数
GLfloat ortho_left = -2.0f;
GLfloat ortho_right = 2.0f;
GLfloat ortho_bottom = -2.0f;
GLfloat ortho_top = 2.0f;
GLfloat ortho_near = -2.0f;
GLfloat ortho_far = 2.0f;
GLfloat persp_fovy = 60.0f;
GLfloat persp_aspect = 1.0f;
GLfloat persp_near = 1.0f;
GLfloat persp_far = 10.0f;
// 绘制立方体
void draw_cube() {
glEnableClientState(GL_VERTEX_ARRAY);
glVertexPointer(3, GL_FLOAT, 0, vertices);
glDrawElements(GL_QUADS, 24, GL_UNSIGNED_BYTE, indices);
glDisableClientState(GL_VERTEX_ARRAY);
}
// 绘制正交投影视图
void draw_ortho_view() {
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(ortho_left, ortho_right, ortho_bottom, ortho_top, ortho_near, ortho_far);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(0.0f, 0.0f, 2.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f);
draw_cube();
}
// 绘制透视投影视图
void draw_persp_view() {
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(persp_fovy, persp_aspect, persp_near, persp_far);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
gluLookAt(0.0f, 0.0f, 4.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f);
draw_cube();
}
// 显示函数
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// 绘制正交投影视图
glViewport(0, 0, 200, 200);
draw_ortho_view();
// 绘制透视投影视图
glViewport(200, 0, 200, 200);
draw_persp_view();
glutSwapBuffers();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(400, 200);
glutCreateWindow("OpenGL Cube");
glClearColor(1.0f, 1.0f, 1.0f, 0.0f);
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
在上述代码中,首先定义了立方体的顶点坐标和面索引。然后定义了正交投影和透视投影的视角参数。接着实现了一个绘制立方体的函数 `draw_cube()`。最后在 `display()` 函数中,使用 `glViewport()` 函数指定窗口区域,分别调用 `draw_ortho_view()` 和 `draw_persp_view()` 函数来绘制正交投影和透视投影视图。其中,通过 `glMatrixMode()` 和 `glLoadIdentity()` 函数来重置投影矩阵和模型视图矩阵,并使用 `glOrtho()` 和 `gluPerspective()` 函数来设置正交投影和透视投影的视角参数。通过 `gluLookAt()` 函数来设置相机位置和目标点位置。最后,通过 `glClear()` 函数清空颜色缓存,并使用 `glutSwapBuffers()` 函数交换前后缓冲区。
阅读全文
相关推荐
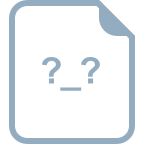




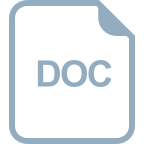
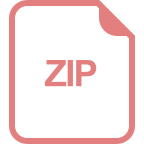
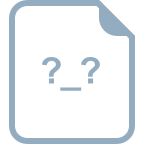
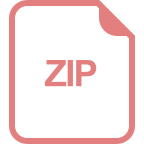
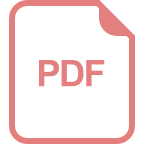
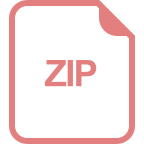