打印二叉树的路径跟祖先,用c语言
时间: 2024-12-15 17:14:40 浏览: 10
要打印二叉树的路径(从根到某个特定节点)以及其最低公共祖先(LCA),你可以按照以下步骤使用C语言:
1. **定义结构体表示二叉树节点**[^1]:
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
```
2. **找到最低公共祖先**:
使用递归方法,对于任意两个给定节点`p`和`q`,如果它们都在同一子树中,那么它们的LCA就是该子树的根;否则,LCA在两者的父节点中,因为它们各自的父亲在不同的子树里。
```c
struct TreeNode* lowestCommonAncestor(struct TreeNode* root, struct TreeNode* p, struct TreeNode* q) {
if (!root || root == p || root == q) return root;
struct TreeNode* leftLca = lowestCommonAncestor(root->left, p, q);
struct TreeNode* rightLca = lowestCommonAncestor(root->right, p, q);
return leftLca && rightLca ? root : leftLca ? leftLca : rightLca;
}
```
3. **打印路径**[^2]:
有了LCA之后,可以遍历从根到LCA的路径。这里以`p`和`q`为例,假设`p`在`q`的左上方,路径可能如`p -> LCA`:
- 对于`p`,如果它不是LCA,就继续在其左侧寻找;
- 当找到LCA时,记录路径并回溯到上一级,直到到达根节点。
```c
void printPathToAncestor(struct TreeNode* node, struct TreeNode* target, vector<int>& path) {
if (node == nullptr) return;
path.push_back(node->val);
if (node == target) {
// 打印路径
for (int i = path.size() - 1; i >= 0; i--) {
printf("%d ", path[i]);
}
printf("\n");
} else {
printPathToAncestor(node->left, target, path);
printPathToAncestor(node->right, target, path);
}
}
```
要调用这些函数,只需传入树的根节点、需要查找路径的节点和LCA即可。
阅读全文
相关推荐















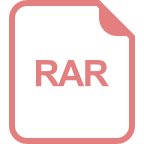



