A*算法编程 (1)任选语言,完成A*算法的实现。 (2)尝试设计场景测试A*算法。
时间: 2024-10-15 22:01:47 浏览: 45
A*算法是一种启发式搜索算法,常用于求解最短路径问题,在游戏开发、地图导航等领域应用广泛。以下是使用Python语言实现一个基本的A*算法的示例:
```python
import heapq
def heuristic(a, b):
# 这里假设两点之间的曼哈顿距离作为启发函数
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def a_star_search(graph, start, goal):
frontier = [(0, start)]
came_from = {}
cost_so_far = {start: 0}
while frontier:
_, current = heapq.heappop(frontier)
if current == goal:
break
for next in graph.neighbors(current):
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
came_from[next] = current
return came_from, cost_so_far
# 示例图数据结构,你可以替换为你需要的数据结构
class Node:
def __init__(self, pos, neighbors, cost=0):
self.pos = pos
self.neighbors = neighbors
self.cost = cost
# 创建图实例并设置起点和终点
graph = Graph([(0, 0), (1, 0), (2, 0)], {(0, 0): [Node((1, 0)), Node((2, 0))], ...})
start = Node((0, 0))
goal = Node((3, 3))
came_from, _ = a_star_search(graph, start, goal)
```
为了测试A*算法,可以设计一些场景,比如在一个迷宫环境中找到从起点到终点的最优路径。首先创建一个二维数组表示迷宫(空格代表通行区域,障碍物代表墙壁),然后使用A*算法计算出一条从起点到终点的路径。
阅读全文
相关推荐
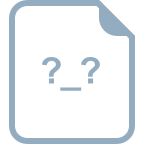
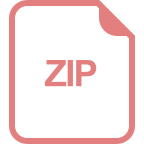
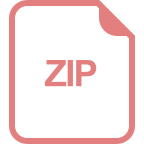












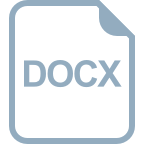