计算轮廓中心 c++
时间: 2023-06-29 08:15:35 浏览: 185
计算轮廓中心的步骤如下:
1. 使用 OpenCV 中的 findContours 函数找到轮廓。
2. 对每个轮廓使用 moments 函数计算其矩,并计算出轮廓的中心点。
3. 如果需要,可以绘制出轮廓及其中心点。
以下是一个简单的 C++ 代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 读取图像并转换为灰度图
Mat src = imread("contours.jpg");
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 二值化图像
Mat binary;
threshold(gray, binary, 0, 255, THRESH_BINARY | THRESH_OTSU);
// 查找轮廓
vector<vector<Point>> contours;
findContours(binary, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 计算每个轮廓的中心点并绘制出来
Mat drawing = Mat::zeros(binary.size(), CV_8UC3);
for (size_t i = 0; i < contours.size(); i++)
{
// 计算轮廓的矩
Moments m = moments(contours[i]);
// 计算轮廓的中心点
Point center(m.m10 / m.m00, m.m01 / m.m00);
// 在图像上绘制出轮廓及其中心点
drawContours(drawing, contours, i, Scalar(0, 0, 255), 2);
circle(drawing, center, 5, Scalar(0, 255, 0), -1);
}
// 显示结果
imshow("Contours", drawing);
waitKey(0);
return 0;
}
```
在这个示例中,我们首先读取一张图像并将其转换为灰度图,然后对其进行二值化处理。接着使用 findContours 函数查找轮廓,并对每个轮廓使用 moments 函数计算其矩并计算出中心点,最后在图像上绘制出轮廓及其中心点。
阅读全文
相关推荐
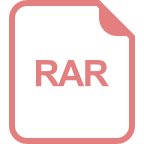









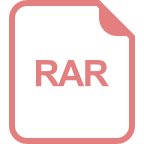
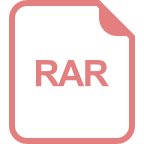
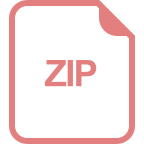


