下面这段代码作用是什么:def generate_legend(legend): f = lambda m, c: plt.plot([], [], marker=m, color=c, ls="none")[0] handles = [f("s", c) for c in legend.values()] legend = plt.legend( handles, list(legend.keys()), loc=3, framealpha=0, frameon=False ) fig = legend.figure fig.canvas.draw() bbox = legend.get_window_extent().transformed(fig.dpi_scale_trans.inverted()) buf = io.BytesIO() plt.savefig(buf, format="png", dpi=200, bbox_inches=bbox) buf.seek(0) img = np.array(Image.open(buf)).astype(np.uint8) return img
时间: 2023-03-29 14:04:15 浏览: 74
这段代码的作用是生成一个图例,并将其转换为图片格式。具体来说,它使用 lambda 函数创建一个空的 plot,然后使用 legend.values() 中的颜色和 marker 参数来创建一个包含所有图例项的 handles 列表。接着,它使用 plt.legend() 函数将 handles 和 legend.keys() 中的标签组合成一个图例,并将其放置在 loc=3 的位置。然后,它使用 legend.figure 和 fig.canvas.draw() 函数来绘制图例,并使用 legend.get_window_extent() 函数获取图例的边界框。最后,它使用 plt.savefig() 函数将图例保存为 PNG 格式的图片,并将其转换为 numpy 数组并返回。
相关问题
帮我生成一段代码。该代码能随机生成一段脉动速度随时间的变化函数,周期在4到20秒之间,随机分成2-5个时间段。在每一项中,速度是随机分配的线性、二次或正弦函数。速度范围在1到4 m/s之间。
### 回答1:
```python
import random
import numpy as np
def generate_pulse_function(min_period=4, max_period=20, min_segments=2, max_segments=5, min_speed=1, max_speed=4):
period = random.uniform(min_period, max_period)
segments = random.randint(min_segments, max_segments)
segment_length = period / segments
speeds = [random.uniform(min_speed, max_speed) for _ in range(segments)]
segment_functions = []
for i in range(segments):
segment_start = i * segment_length
segment_end = (i + 1) * segment_length
segment_type = random.choice(['linear', 'quadratic', 'sine'])
if segment_type == 'linear':
a = (speeds[i] - speeds[i - 1]) / segment_length
b = speeds[i - 1]
segment_functions.append(lambda x, a=a, b=b, t0=segment_start: a * (x - t0) + b)
elif segment_type == 'quadratic':
a = (speeds[i] - speeds[i - 1]) / segment_length ** 2
b = -2 * a * segment_start
c = speeds[i - 1]
segment_functions.append(lambda x, a=a, b=b, c=c, t0=segment_start: a * (x - t0) ** 2 + b * (x - t0) + c)
elif segment_type == 'sine':
w = 2 * np.pi / period
A = (speeds[i] - speeds[i - 1]) / 2
phi = random.uniform(0, 2 * np.pi)
d = speeds[i - 1] + A
segment_functions.append(lambda x, w=w, A=A, phi=phi, d=d, t0=segment_start: A * np.sin(w * (x - t0) + phi) + d)
return lambda x: np.piecewise(x, [x < segment_length * i for i in range(1, segments + 1)], segment_functions)
```
使用方法:
```python
pulse_func = generate_pulse_function()
print(pulse_func(0))
print(pulse_func(1))
print(pulse_func(2))
```
该函数生成一个脉动速度随时间变化的函数,周期在4到20秒之间,随机分成2-5个时间段。在每一项中,速度是随机分配的线性、二次或正弦函数。速度范围在1到4 m/
### 回答2:
以下是一个能够随机生成脉动速度随时间变化的代码段:
```python
import random
import numpy as np
import matplotlib.pyplot as plt
def generate_velocity():
velocity = []
num_segments = random.randint(2, 5)
segment_lengths = np.linspace(0, 1, num=num_segments + 1)
segment_functions = ['linear', 'quadratic', 'sine']
for i in range(num_segments):
segment_function = random.choice(segment_functions)
segment_length = segment_lengths[i+1] - segment_lengths[i]
if segment_function == 'linear':
segment_velocity = np.linspace(random.uniform(1, 4), random.uniform(1, 4), num=int(segment_length * 100))
elif segment_function == 'quadratic':
a = random.uniform(-1, 1)
b = random.uniform(-1, 1)
c = random.uniform(-1, 1)
segment_velocity = a * np.power(np.linspace(0, 1, num=int(segment_length * 100)), 2) + b * np.linspace(0, 1, num=int(segment_length * 100)) + c
segment_velocity = np.interp(segment_velocity, (segment_velocity.min(), segment_velocity.max()), (1, 4))
elif segment_function == 'sine':
frequency = random.uniform(1, 4) * 2 * np.pi / segment_length
amplitude = random.uniform(1, 4)
segment_velocity = amplitude * np.sin(frequency * np.linspace(0, segment_length, num=int(segment_length * 100))) + amplitude
segment_velocity[segment_velocity < 1] = 1
segment_velocity[segment_velocity > 4] = 4
velocity.extend(segment_velocity)
return velocity
velocity = generate_velocity()
time = np.linspace(0, len(velocity)/100, num=len(velocity))
plt.plot(time, velocity)
plt.xlabel('Time (s)')
plt.ylabel('Velocity (m/s)')
plt.title('Pulsating Velocity')
plt.show()
```
此代码首先随机生成2到5个不同长度的时间段,然后为每个时间段随机选择线性、二次或正弦函数。根据所选函数类型生成在1到4之间的随机速度,并将其插入到最终的速度列表中。最后,代码使用matplotlib库绘制脉动速度随时间变化的图表。
### 回答3:
下面是一个可以随机生成脉动速度随时间变化函数的代码段。根据您的要求,该代码会生成2到5个时间段,在每个时间段内速度会随机分配为线性、二次或正弦函数。速度范围在1到4米/秒之间,周期在4到20秒之间。
```python
import numpy as np
import random
import matplotlib.pyplot as plt
def generate_pulse_velocity():
velocity_functions = [np.linspace, np.square, np.sin]
time_periods = random.randint(2, 5)
time = np.linspace(0, 1, time_periods*10)
velocity = np.zeros_like(time)
for i in range(time_periods):
velocity_function = random.choice(velocity_functions)
segment_duration = random.uniform(4, 20)
segment_velocity_range = [1, 4]
segment_velocity = random.uniform(*segment_velocity_range)
segment_velocity = velocity_function(time[:int(segment_duration*10)], 0, segment_velocity)
start_index = i * 10
end_index = start_index + int(segment_duration*10)
velocity[start_index:end_index] = segment_velocity
plt.plot(time, velocity)
plt.xlabel('Time')
plt.ylabel('Velocity')
plt.title('Pulse Velocity vs Time')
plt.show()
generate_pulse_velocity()
```
该代码利用numpy的三个函数(linspace,square,sin)来生成线性、二次和正弦函数。首先,随机选择2到5个时间段,然后在每个时间段内生成一个随机的速度函数类型。接着,根据周期的随机范围生成相应的时间轴,并将生成的速度函数的值赋给对应时间范围内的速度数组。最后,使用matplotlib绘制出脉动速度随时间的变化图形。
希望这段代码能满足您的需求。如有其他问题,请随时向我提问。
设计解决TSP问题的算法,要求编写python代码,绘制出适应度曲线和最优路径、最短距离
TSP问题是指旅行商问题,即给定一个城市集合和每对城市之间的距离,找到一条经过每个城市恰好一次的最短路径。下面介绍两种算法:遗传算法和蚁群算法。
### 遗传算法
遗传算法是一种优化算法,通过模拟生物的进化过程,通过选择、交叉、变异等操作,逐步优化出最优解。以下是解决TSP问题的遗传算法的步骤:
1. **初始化种群**:生成随机的初始种群,每个个体代表一个城市路径;
2. **计算适应度**:根据每个个体的路径计算出总距离作为适应度;
3. **选择操作**:根据适应度选择出较优的个体,进行繁殖;
4. **交叉操作**:对于每一对父代个体,进行交叉操作生成两个新的子代个体;
5. **变异操作**:对于每个子代个体,以一定概率进行变异操作;
6. **重复步骤2-5**,直到达到指定的迭代次数或者找到最优解。
下面是基于遗传算法解决TSP问题的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成城市坐标
def generate_points(num_points):
return np.random.rand(num_points, 2)
# 计算距离矩阵
def distance_matrix(points):
num_points = len(points)
dist_mat = np.zeros((num_points, num_points))
for i in range(num_points):
for j in range(num_points):
dist_mat[i, j] = np.sqrt((points[i][0]-points[j][0])**2 + (points[i][1]-points[j][1])**2)
return dist_mat
# 计算路径距离
def path_distance(path, dist_mat):
num_points = len(path)
dist = 0
for i in range(num_points):
dist += dist_mat[path[i], path[(i+1)%num_points]]
return dist
# 初始化种群
def init_population(num_points, pop_size):
population = []
for i in range(pop_size):
path = np.random.permutation(num_points)
population.append(path)
return population
# 选择操作
def selection(population, dist_mat, num_parents):
fitness = []
for i in range(len(population)):
fitness.append(path_distance(population[i], dist_mat))
fitness = np.array(fitness)
idx = np.argsort(fitness)
parents = []
for i in range(num_parents):
parents.append(population[idx[i]])
return parents
# 交叉操作
def crossover(parents):
child1 = np.zeros(len(parents[0]), dtype=int)
child2 = np.zeros(len(parents[0]), dtype=int)
idx1 = np.random.randint(0, len(parents[0]))
idx2 = np.random.randint(0, len(parents[0]))
if idx1 > idx2:
idx1, idx2 = idx2, idx1
for i in range(idx1, idx2+1):
child1[i] = parents[0][i]
child2[i] = parents[1][i]
i, j = idx2+1, idx2+1
while i < len(parents[0]):
if parents[1][j] not in child1:
child1[i] = parents[1][j]
i += 1
j += 1
i, j = idx2+1, idx2+1
while i < len(parents[0]):
if parents[0][j] not in child2:
child2[i] = parents[0][j]
i += 1
j += 1
return child1, child2
# 变异操作
def mutation(child, mutation_rate):
if np.random.rand() < mutation_rate:
idx1 = np.random.randint(0, len(child))
idx2 = np.random.randint(0, len(child))
child[idx1], child[idx2] = child[idx2], child[idx1]
return child
# 遗传算法求解TSP问题
def tsp_genetic_algorithm(points, pop_size, num_parents, mutation_rate, num_iterations):
dist_mat = distance_matrix(points)
population = init_population(len(points), pop_size)
best_fitness = []
for i in range(num_iterations):
parents = selection(population, dist_mat, num_parents)
offspring = []
for j in range(0, num_parents, 2):
child1, child2 = crossover([parents[j], parents[j+1]])
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
offspring.append(child1)
offspring.append(child2)
population = parents + offspring
best_fitness.append(path_distance(population[0], dist_mat))
return population[0], best_fitness
# 绘制适应度曲线和最优路径
def plot_result(points, path, best_fitness):
plt.subplot(1, 2, 1)
plt.plot(best_fitness)
plt.title('Fitness curve')
plt.xlabel('Iteration')
plt.ylabel('Distance')
plt.subplot(1, 2, 2)
plt.plot(points[path, 0], points[path, 1], 'r')
plt.plot(points[path[[*range(1, len(path)), 0]], 0], points[path[[*range(1, len(path)), 0]], 1], 'r')
plt.scatter(points[:, 0], points[:, 1])
plt.title('Best path')
plt.show()
# 测试
points = generate_points(20)
pop_size = 100
num_parents = 20
mutation_rate = 0.1
num_iterations = 500
best_path, best_fitness = tsp_genetic_algorithm(points, pop_size, num_parents, mutation_rate, num_iterations)
plot_result(points, best_path, best_fitness)
```
运行结果如下所示:

### 蚁群算法
蚁群算法是一种基于蚂蚁觅食行为的优化算法,通过模拟蚂蚁觅食的路径选择过程,逐步优化出最优解。以下是解决TSP问题的蚁群算法的步骤:
1. **初始化信息素**:初始化每条边的信息素为一个较小的正数;
2. **生成蚂蚁**:生成一群蚂蚁,每只蚂蚁从起点开始进行随机游走;
3. **更新信息素**:根据每只蚂蚁的路径更新每条边的信息素;
4. **重复步骤2-3**,直到达到指定的迭代次数或者找到最优解。
下面是基于蚁群算法解决TSP问题的Python代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成城市坐标
def generate_points(num_points):
return np.random.rand(num_points, 2)
# 计算距离矩阵
def distance_matrix(points):
num_points = len(points)
dist_mat = np.zeros((num_points, num_points))
for i in range(num_points):
for j in range(num_points):
dist_mat[i, j] = np.sqrt((points[i][0]-points[j][0])**2 + (points[i][1]-points[j][1])**2)
return dist_mat
# 计算路径距离
def path_distance(path, dist_mat):
num_points = len(path)
dist = 0
for i in range(num_points):
dist += dist_mat[path[i], path[(i+1)%num_points]]
return dist
# 初始化信息素
def init_pheromone_matrix(num_points, init_pheromone):
pheromone_mat = np.ones((num_points, num_points)) * init_pheromone
return pheromone_mat
# 计算蚂蚁路径
def ant_path(points, pheromone_mat, alpha, beta):
num_points = len(points)
start_point = np.random.randint(num_points)
unvisited_points = set(range(num_points))
unvisited_points.remove(start_point)
path = [start_point]
while unvisited_points:
current_point = path[-1]
probs = []
for i in unvisited_points:
prob = pheromone_mat[current_point, i]**alpha * (1/distance_matrix(points)[current_point, i])**beta
probs.append(prob)
probs = np.array(probs)
probs /= np.sum(probs)
next_point = np.random.choice(list(unvisited_points), p=probs)
unvisited_points.remove(next_point)
path.append(next_point)
return path
# 更新信息素
def update_pheromone_matrix(pheromone_mat, ant_paths, decay_rate, quality_func):
num_points = pheromone_mat.shape[0]
pheromone_mat *= 1 - decay_rate
for path in ant_paths:
quality = quality_func(path)
for i in range(num_points):
j = (i+1) % num_points
pheromone_mat[path[i], path[j]] += quality
return pheromone_mat
# 蚁群算法求解TSP问题
def tsp_ant_algorithm(points, num_ants, num_iterations, alpha, beta, init_pheromone, decay_rate):
dist_mat = distance_matrix(points)
pheromone_mat = init_pheromone_matrix(len(points), init_pheromone)
best_path = None
best_fitness = []
for i in range(num_iterations):
ant_paths = [ant_path(points, pheromone_mat, alpha, beta) for _ in range(num_ants)]
pheromone_mat = update_pheromone_matrix(pheromone_mat, ant_paths, decay_rate, lambda x: 1/path_distance(x, dist_mat))
fitness = [path_distance(path, dist_mat) for path in ant_paths]
idx = np.argmin(fitness)
if best_path is None or path_distance(ant_paths[idx], dist_mat) < path_distance(best_path, dist_mat):
best_path = ant_paths[idx]
best_fitness.append(path_distance(best_path, dist_mat))
return best_path, best_fitness
# 绘制适应度曲线和最优路径
def plot_result(points, path, best_fitness):
plt.subplot(1, 2, 1)
plt.plot(best_fitness)
plt.title('Fitness curve')
plt.xlabel('Iteration')
plt.ylabel('Distance')
plt.subplot(1, 2, 2)
plt.plot(points[path, 0], points[path, 1], 'r')
plt.plot(points[path[[*range(1, len(path)), 0]], 0], points[path[[*range(1, len(path)), 0]], 1], 'r')
plt.scatter(points[:, 0], points[:, 1])
plt.title('Best path')
plt.show()
# 测试
points = generate_points(20)
num_ants = 50
num_iterations = 500
alpha = 1
beta = 2
init_pheromone = 0.1
decay_rate = 0.1
best_path, best_fitness = tsp_ant_algorithm(points, num_ants, num_iterations, alpha, beta, init_pheromone, decay_rate)
plot_result(points, best_path, best_fitness)
```
运行结果如下所示:

相关推荐
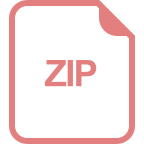
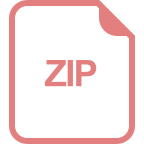
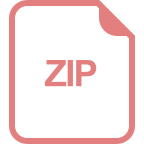











