我们人类习惯于书写“中缀式”,如 3 + 5 * 2 ,其值为13。 (p.s. 为什么人类习惯中缀式呢?是因为中缀式比后缀式好用么?) 而计算机更加习惯“后缀式”(也叫“逆波兰式”,Reverse Polish Notation)。上述中缀式对应的后缀式是: 3 5 2 * + 现在,请对输入的后缀式进行求值。 输入格式: 在一行中输入一个后缀式,运算数和运算符之间用空格分隔,运算数长度不超过6位,运算符仅有+ - * / 四种。 输出格式: 在一行中输出后缀式的值,保留一位小数。 输入样例: 3 5.4 2.2 * + 输出样例: 14.9 代码长度限制 16 KB 时间限制 400 ms 内存限制 64 MB 用 C 语言回答
时间: 2024-03-24 08:38:41 浏览: 156
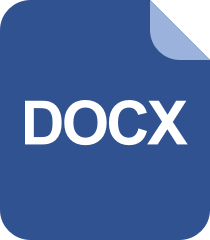
数据结构实验报告-栈与队列-中缀表达式转换为后缀式5分-实验内容及要求.docx

以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100 // 后缀式中运算数和运算符的最大数量
// 定义栈结构
typedef struct {
double data[MAX_SIZE]; // 存储栈中元素的数组
int top; // 栈顶指针
} Stack;
// 初始化栈
void initStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack *s) {
return s->top == MAX_SIZE - 1;
}
// 入栈
void push(Stack *s, double x) {
if (isFull(s)) {
printf("Error: Stack is full!\n");
exit(EXIT_FAILURE);
}
s->data[++(s->top)] = x;
}
// 出栈
double pop(Stack *s) {
if (isEmpty(s)) {
printf("Error: Stack is empty!\n");
exit(EXIT_FAILURE);
}
return s->data[(s->top)--];
}
int main() {
Stack s; // 定义一个栈
initStack(&s); // 初始化栈
char str[MAX_SIZE]; // 存储后缀式的字符串
fgets(str, MAX_SIZE, stdin); // 从标准输入读入后缀式字符串
int len = strlen(str); // 后缀式字符串的长度
double num; // 存储运算数的变量
for (int i = 0; i < len; i++) {
if (str[i] == ' ') { // 遇到空格则跳过
continue;
} else if (str[i] >= '0' && str[i] <= '9') { // 遇到数字则转为double类型后入栈
num = 0;
while (str[i] >= '0' && str[i] <= '9' || str[i] == '.') {
if (str[i] != '.') {
num = num * 10 + str[i] - '0';
} else {
double decimal = 0.1;
while (str[++i] >= '0' && str[i] <= '9') {
num += (str[i] - '0') * decimal;
decimal *= 0.1;
}
break;
}
i++;
}
push(&s, num);
} else { // 遇到运算符则从栈中取出相应数量的运算数进行计算,并将计算结果入栈
double num2 = pop(&s);
double num1 = pop(&s);
switch (str[i]) {
case '+':
push(&s, num1 + num2);
break;
case '-':
push(&s, num1 - num2);
break;
case '*':
push(&s, num1 * num2);
break;
case '/':
push(&s, num1 / num2);
break;
default:
printf("Error: Invalid operator!\n");
exit(EXIT_FAILURE);
}
}
}
printf("%.1f\n", pop(&s)); // 栈中剩下的元素即为后缀式的值
return 0;
}
```
阅读全文
相关推荐
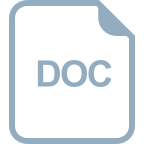
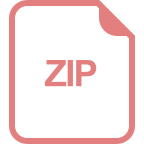















