c++ 保存bmp
时间: 2023-06-28 15:16:26 浏览: 91
要在C++中保存BMP文件,可以使用以下步骤:
1. 定义BMP文件头和图像数据的结构体。BMP文件头包含文件类型、大小等信息,图像数据包含像素点的颜色和位置信息。
2. 初始化BMP文件头和图像数据。
3. 将像素点的颜色和位置信息写入图像数据。
4. 将BMP文件头和图像数据写入文件。
下面是一个简单的示例代码:
```
#include <fstream>
#include <cstdint>
#pragma pack(push, 1) //设置数据结构体的1字节对齐
struct BMPHeader {
uint16_t type{0x4D42}; //文件类型,固定为BM
uint32_t size{0}; //文件大小,包括文件头和图像数据总大小
uint32_t reserved{0}; //保留字段,必须为0
uint32_t offset{0}; //图像数据相对于文件头的偏移量
uint32_t header_size{40}; //信息头大小,固定为40
uint32_t width{0}; //图像宽度,单位为像素
uint32_t height{0}; //图像高度,单位为像素
uint16_t planes{1}; //位面数,必须为1
uint16_t bit_count{24}; //每个像素点的位数,一般为24
uint32_t compression{0}; //压缩方式,0表示不压缩
uint32_t size_image{0}; //图像数据大小,包括填充字节
uint32_t x_pixels_per_meter{0}; //水平分辨率,单位为像素/米
uint32_t y_pixels_per_meter{0}; //垂直分辨率,单位为像素/米
uint32_t colors_used{0}; //使用的颜色数,0表示使用所有调色板
uint32_t colors_important{0}; //重要的颜色数,0表示所有颜色都重要
};
#pragma pack(pop) //取消1字节对齐
void save_bmp(const char* filename, int width, int height, uint8_t* data) {
std::ofstream file(filename, std::ios::binary);
if (!file) {
throw std::runtime_error("Failed to open file for writing");
}
BMPHeader header{};
header.width = width;
header.height = height;
header.size_image = (((header.width * header.bit_count + 31) & ~31) / 8) * header.height;
header.size = sizeof(BMPHeader) + header.size_image;
header.offset = sizeof(BMPHeader);
file.write(reinterpret_cast<const char*>(&header), sizeof(BMPHeader));
int padding = (4 - (header.width * 3) % 4) % 4; //每行补齐字节
for (int y = header.height - 1; y >= 0; --y) { //BMP图像数据从下往上存储
for (int x = 0; x < header.width; ++x) {
uint8_t* pixel = data + (y * header.width + x) * 3;
file.write(reinterpret_cast<const char*>(pixel + 2), 1); //BMP图像的像素顺序是BGR
file.write(reinterpret_cast<const char*>(pixel + 1), 1);
file.write(reinterpret_cast<const char*>(pixel), 1);
}
for (int i = 0; i < padding; ++i) {
file.put(0); //补齐字节
}
}
}
```
使用时,可以调用save_bmp函数,传入文件名、图像宽度、高度和像素数据即可保存BMP文件。
阅读全文
相关推荐
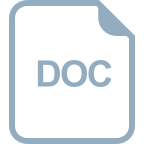
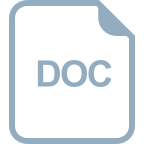
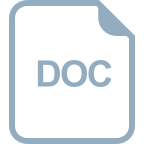

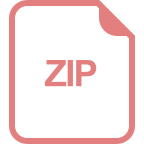
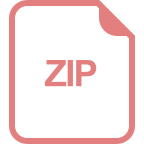
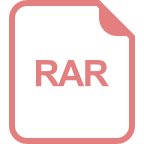
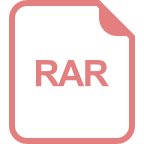
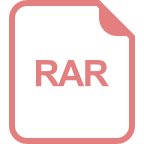
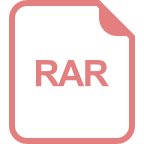
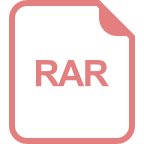
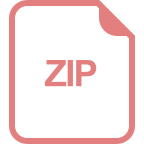
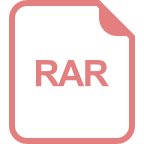
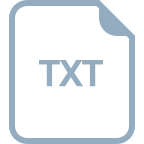
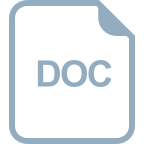



