生成代码 计算第一个三维向量与第二个三维向量之间的夹角,夹角范围在-360~360
时间: 2024-03-23 18:36:08 浏览: 15
以下是 Python 代码示例:
```python
import math
def angle_between_vectors(a, b):
dot_product = a[0]*b[0] + a[1]*b[1] + a[2]*b[2]
magnitude_a = math.sqrt(a[0]**2 + a[1]**2 + a[2]**2)
magnitude_b = math.sqrt(b[0]**2 + b[1]**2 + b[2]**2)
cos_theta = dot_product / (magnitude_a * magnitude_b)
theta = math.acos(cos_theta)
if math.isnan(theta):
return 0.0
else:
return math.degrees(theta)
# Example usage
a = (1, 2, 3)
b = (4, 5, 6)
theta = angle_between_vectors(a, b)
print(theta)
```
这个函数接受两个三维向量作为输入,并返回它们之间的夹角,夹角的范围在 -360 到 360 度之间。如果两个向量重合,夹角为 0 度。
相关问题
c语言/c++生成代码 计算第一个三维向量与第二个三维向量之间的夹角,夹角范围在-360~360
以下是 C++ 代码示例:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
double angle_between_vectors(double a[], double b[]) {
double dot_product = a[0]*b[0] + a[1]*b[1] + a[2]*b[2];
double magnitude_a = sqrt(a[0]*a[0] + a[1]*a[1] + a[2]*a[2]);
double magnitude_b = sqrt(b[0]*b[0] + b[1]*b[1] + b[2]*b[2]);
double cos_theta = dot_product / (magnitude_a * magnitude_b);
double theta = acos(cos_theta);
if (isnan(theta)) {
return 0.0;
} else {
return theta * 180.0 / M_PI;
}
}
// Example usage
int main() {
double a[3] = {1, 2, 3};
double b[3] = {4, 5, 6};
double theta = angle_between_vectors(a, b);
cout << theta << endl;
return 0;
}
```
这个函数接受两个三维向量作为输入,并返回它们之间的夹角,夹角的范围在 -360 到 360 度之间。如果两个向量重合,夹角为 0 度。注意,C++ 中的 `acos()` 函数返回的是弧度值,因此需要将其乘以 180/π 转换为角度值。
c++求两个三维向量求夹角代码
可以使用向量的点积和模长公式来求解两个三维向量的夹角。
假设有两个向量 a 和 b,则它们的夹角 θ 可以用以下公式计算:
cosθ = (a·b) / (|a| × |b|)
其中,· 表示向量的点积(即各个对应分量相乘之和),|a| 和 |b| 分别表示向量 a 和 b 的模长。
则两个向量的夹角为:
θ = acos((a·b) / (|a| × |b|))
下面是一个简单的 C 代码实现:
```c
#include <stdio.h>
#include <math.h>
typedef struct {
double x, y, z;
} Vector3;
// 计算向量模长
double length(Vector3 v) {
return sqrt(v.x * v.x + v.y * v.y + v.z * v.z);
}
// 计算向量点积
double dot(Vector3 a, Vector3 b) {
return a.x * b.x + a.y * b.y + a.z * b.z;
}
// 计算向量夹角(单位:弧度)
double angle(Vector3 a, Vector3 b) {
double cosTheta = dot(a, b) / (length(a) * length(b));
return acos(cosTheta);
}
int main() {
Vector3 a = {1, 2, 3};
Vector3 b = {4, 5, 6};
double theta = angle(a, b);
printf("The angle between a and b is %.2f radians.\n", theta);
return 0;
}
```
注意:上述代码中的向量夹角是以弧度为单位的。如果需要以角度为单位,则需要将弧度值乘以 180 / π。
相关推荐
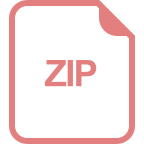
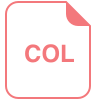
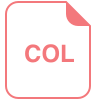










