c++实现求两个三维向量的夹角
时间: 2024-05-13 17:16:36 浏览: 193
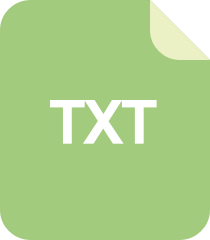
以知2条向量求向量得夹角

可以通过向量的点积和向量模长的乘积计算两个向量的夹角。具体实现如下:
```c++
#include <iostream>
#include <cmath>
using namespace std;
struct Vector3 {
double x, y, z;
Vector3(double _x, double _y, double _z): x(_x), y(_y), z(_z) {}
};
double dotProduct(const Vector3& a, const Vector3& b) {
return a.x * b.x + a.y * b.y + a.z * b.z;
}
double vectorLength(const Vector3& v) {
return sqrt(v.x * v.x + v.y * v.y + v.z * v.z);
}
double vectorAngle(const Vector3& a, const Vector3& b) {
double cosAngle = dotProduct(a, b) / (vectorLength(a) * vectorLength(b));
return acos(cosAngle);
}
int main() {
Vector3 a(1, 2, 3);
Vector3 b(4, 5, 6);
double angle = vectorAngle(a, b);
cout << "The angle between a and b is " << angle << " radians." << endl;
return 0;
}
```
其中,`Vector3` 结构体存储三维向量的坐标,`dotProduct` 函数计算两个向量的点积,`vectorLength` 函数计算向量的模长,`vectorAngle` 函数计算两个向量的夹角,最后在 `main` 函数中测试实现的正确性。
阅读全文
相关推荐

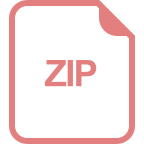
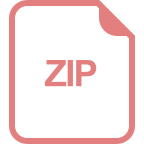




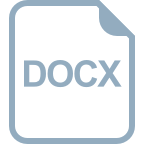
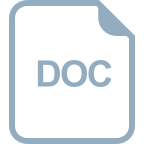
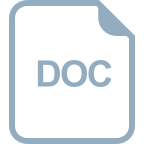




