用c++计算三维点之间夹角
时间: 2024-02-25 22:56:37 浏览: 34
可以使用向量的点积公式来计算三维点之间的夹角,具体实现如下(假设有三个点A(x1,y1,z1)、B(x2,y2,z2)、C(x3,y3,z3)):
```c++
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double x1, y1, z1, x2, y2, z2, x3, y3, z3;
cout << "请输入三个点的坐标(x,y,z):" << endl;
cin >> x1 >> y1 >> z1 >> x2 >> y2 >> z2 >> x3 >> y3 >> z3;
// 计算向量AB和向量AC
double vectorAB[3] = {x2 - x1, y2 - y1, z2 - z1};
double vectorAC[3] = {x3 - x1, y3 - y1, z3 - z1};
// 计算向量AB和向量AC的点积
double dotProduct = vectorAB[0] * vectorAC[0] + vectorAB[1] * vectorAC[1] + vectorAB[2] * vectorAC[2];
// 计算向量AB和向量AC的模长
double moduleAB = sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2) + pow(z2 - z1, 2));
double moduleAC = sqrt(pow(x3 - x1, 2) + pow(y3 - y1, 2) + pow(z3 - z1, 2));
// 计算向量AB和向量AC的夹角的余弦值
double cosine = dotProduct / (moduleAB * moduleAC);
// 计算向量AB和向量AC的夹角的弧度值
double radian = acos(cosine);
// 将弧度值转换为角度值
double angle = radian * 180.0 / M_PI;
cout << "三个点之间的夹角为:" << angle << "度" << endl;
return 0;
}
```
其中,M_PI为圆周率,需要在代码中包含math.h头文件。
相关推荐
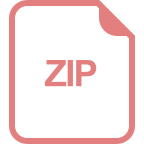
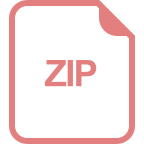














