Hadoop编写实现矩阵相乘功能
时间: 2023-12-25 11:57:43 浏览: 27
Hadoop是一个分布式计算框架,可以用于处理大规模数据。矩阵相乘是一个经典的计算问题,也可以使用Hadoop来解决。下面是一个简单的Hadoop实现矩阵相乘的示例:
1. 输入数据的准备
首先,我们需要准备两个矩阵A和B,它们的维度分别为m x k和k x n。我们将它们存储在Hadoop分布式文件系统(HDFS)中,以便可以在Hadoop集群的所有节点上访问它们。
2. Map阶段
在Map阶段,我们需要将矩阵A和矩阵B分别读入内存,并进行分块处理。我们将矩阵A分成m个子矩阵,每个子矩阵的大小为1 x k;将矩阵B分成n个子矩阵,每个子矩阵的大小为k x 1。然后,对于每个子矩阵,我们将其作为键值对的键,将其对应的行或列作为值,发送给Reducer节点。
3. Reduce阶段
在Reduce阶段,我们需要将来自Map节点的键值对进行合并,并进行矩阵相乘操作。具体来说,对于每个子矩阵A和子矩阵B,我们需要将它们相乘,并将结果累加到最终的结果矩阵C中。最后,我们将结果矩阵C输出到HDFS中。
下面是一个简单的Hadoop程序,用于实现矩阵相乘功能:
```java
public class MatrixMultiplication {
public static class MapClass extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
// 读入矩阵A和矩阵B
...
// 将矩阵A分块处理,并将每个子矩阵作为键值对的键,将其对应的行作为值发送给Reducer节点
for (int i = 0; i < m; i++) {
for (int j = 0; j < k; j++) {
Text outKey = new Text(i + "," + j);
Text outValue = new Text("A," + j + "," + A[i][j]);
context.write(outKey, outValue);
}
}
// 将矩阵B分块处理,并将每个子矩阵作为键值对的键,将其对应的列作为值发送给Reducer节点
for (int i = 0; i < k; i++) {
for (int j = 0; j < n; j++) {
Text outKey = new Text(i + "," + j);
Text outValue = new Text("B," + i + "," + B[i][j]);
context.write(outKey, outValue);
}
}
}
}
public static class ReduceClass extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// 将来自Map节点的键值对进行合并,并进行矩阵相乘操作
...
// 将结果矩阵C输出到HDFS中
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
Text outKey = new Text(i + "," + j);
Text outValue = new Text("" + C[i][j]);
context.write(outKey, outValue);
}
}
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = new Job(conf, "MatrixMultiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(MapClass.class);
job.setReducerClass(ReduceClass.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在上面的程序中,我们首先定义了一个MapClass和一个ReduceClass,分别用于执行Map阶段和Reduce阶段的操作。然后,我们在main函数中配置了作业,并将其提交给Hadoop进行执行。
在实际使用中,我们需要将矩阵A和矩阵B存储在HDFS中,并指定输入路径和输出路径。我们可以使用以下命令运行程序:
```bash
hadoop jar matrixmultiplication.jar MatrixMultiplication input output
```
其中,input是存储矩阵A和矩阵B的输入路径,output是存储结果矩阵C的输出路径。
相关推荐
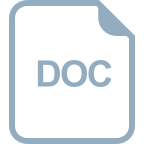













