hadoop编写矩阵相乘
时间: 2023-11-14 20:14:05 浏览: 139
在 Hadoop 中,矩阵相乘可以使用 MapReduce 实现。具体步骤如下:
1. Map 阶段:将两个矩阵中的元素映射为键值对,其中键表示元素所在的行和列,值表示元素的值。
2. Reduce 阶段:将相同键的值相乘并相加,得到相乘结果。
下面是 Hadoop 中矩阵相乘的代码实现:
```java
public class MatrixMultiplication {
public static class MatrixMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text outputKey = new Text();
private Text outputValue = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] line = value.toString().split(",");
if (line[0].equals("A")) {
for (int i = 0; i < Integer.parseInt(context.getConfiguration().get("n")); i++) {
outputKey.set(line[1] + "," + i);
outputValue.set("A," + line[2] + "," + line[3]);
context.write(outputKey, outputValue);
}
} else {
for (int i = 0; i < Integer.parseInt(context.getConfiguration().get("p")); i++) {
outputKey.set(i + "," + line[3]);
outputValue.set("B," + line[1] + "," + line[2]);
context.write(outputKey, outputValue);
}
}
}
}
public static class MatrixReducer extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
int[] a = new int[Integer.parseInt(context.getConfiguration().get("m"))];
int[] b = new int[Integer.parseInt(context.getConfiguration().get("p"))];
for (Text value : values) {
String[] line = value.toString().split(",");
if (line[0].equals("A")) {
a[Integer.parseInt(line[1])] = Integer.parseInt(line[2]);
} else {
b[Integer.parseInt(line[1])] = Integer.parseInt(line[2]);
}
}
int sum = 0;
for (int i = 0; i < a.length; i++) {
sum += a[i] * b[i];
}
context.write(key, new Text(Integer.toString(sum)));
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
conf.set("m", args[0]);
conf.set("n", args[1]);
conf.set("p", args[2]);
Job job = Job.getInstance(conf, "Matrix Multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[3]));
FileOutputFormat.setOutputPath(job, new Path(args[4]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在运行时,需要指定三个参数,分别为矩阵 A 的行数 m、矩阵 B 的列数 p,以及矩阵 A 和 B 的输入文件路径。例如:
```shell
$ hadoop jar MatrixMultiplication.jar 2 3 2 input/A.txt input/B.txt output
```
其中,A.txt 和 B.txt 文件格式如下:
A.txt:
```
A,0,0,1
A,0,1,2
A,1,0,3
A,1,1,4
```
B.txt:
```
B,0,0,5
B,1,0,6
B,2,0,7
B,0,1,8
B,1,1,9
B,2,1,10
```
输出结果会保存在 output 目录下。
阅读全文
相关推荐
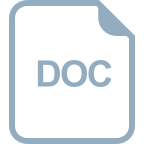
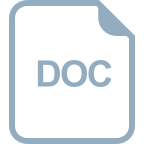
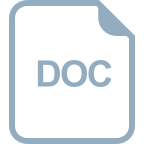









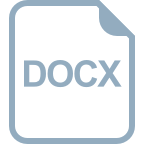




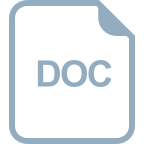
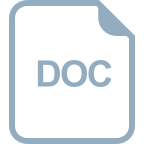