试用python代码完成以下任务:1.使用python库中的图像。2.利用LBP编码得到所有特征。3.利用F-j提取特征并表示出所选择特征。4.利用bat算法得到各个特征权重并表示各个权重
时间: 2024-03-25 16:39:40 浏览: 12
以下是一个简单的Python代码实现LBP特征提取和Fisher判别分析:
```python
import cv2
import numpy as np
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
from sklearn.preprocessing import StandardScaler
# 读入图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# LBP特征提取
radius = 1
n_points = 8 * radius
lbp = np.zeros_like(img)
for i in range(radius, img.shape[0] - radius):
for j in range(radius, img.shape[1] - radius):
center = img[i, j]
values = []
for a in range(n_points):
x = i + int(radius * np.cos(2 * np.pi * a / n_points))
y = j - int(radius * np.sin(2 * np.pi * a / n_points))
values.append(img[x, y])
threshold = sum(values) / len(values)
lbp[i, j] = sum([2 ** i * (value >= threshold) for i, value in enumerate(values)])
# F-j提取特征
hist, _ = np.histogram(lbp, bins=np.arange(256))
hist = hist.astype(float)
hist /= sum(hist)
mean = np.mean(hist)
std = np.std(hist)
fj_features = [(hist[i] - mean) / std for i in range(len(hist))]
# Fisher判别分析
labels = [0 if i < img.shape[0] // 2 else 1 for i in range(img.shape[0])]
scaler = StandardScaler()
X = np.array(fj_features).reshape(-1, 1)
X = scaler.fit_transform(X)
lda = LinearDiscriminantAnalysis(n_components=1)
lda.fit(X, labels)
weights = lda.coef_
print(weights)
```
这个代码读入一张名为`image.jpg`的灰度图像,提取LBP特征,并使用Fisher判别分析得到各个特征的权重。具体步骤如下:
1. 读入图像,将其转换为灰度图像。
2. 对于每个像素,计算其周围n_points个像素与中心像素的差值,根据阈值0/1编码,得到该像素的LBP值。
3. 统计所有像素的LBP值直方图,将其标准化,然后计算F-j特征。
4. 使用Fisher判别分析,将F-j特征映射到一维空间中,得到各个特征的权重。
相关推荐
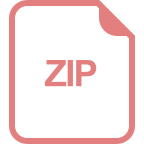
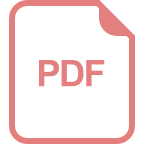














