设计一个三维空间中的向量类,要求如下: (1)向量类的构造函数; (2)用(x,y,z)的形式对三维向量进行输出; (3)用成员函数计算向量的长度; (4)用友元函数计算向量的和与差。 2、编程实现Student类,在该类中包含数据成员分数Score,静态数据成员学生人数count和总分total,设计静态成员函数完成总分和平均值的计算。用c++作答
时间: 2024-02-21 10:01:49 浏览: 88
好的,我来回答您的问题。
首先是第一个问题,设计一个三维空间中的向量类,满足要求如下:
```c++
class Vector3D {
public:
Vector3D(float x, float y, float z);
void print();
float length();
friend Vector3D operator+(const Vector3D& v1, const Vector3D& v2);
friend Vector3D operator-(const Vector3D& v1, const Vector3D& v2);
private:
float m_x;
float m_y;
float m_z;
};
```
其中,构造函数如下:
```c++
Vector3D::Vector3D(float x, float y, float z)
: m_x(x), m_y(y), m_z(z)
{
}
```
输出函数实现如下:
```c++
void Vector3D::print()
{
std::cout << "(" << m_x << ", " << m_y << ", " << m_z << ")" << std::endl;
}
```
长度函数实现如下:
```c++
float Vector3D::length()
{
return sqrt(m_x * m_x + m_y * m_y + m_z * m_z);
}
```
向量的加减法实现如下:
```c++
Vector3D operator+(const Vector3D& v1, const Vector3D& v2)
{
return Vector3D(v1.m_x + v2.m_x, v1.m_y + v2.m_y, v1.m_z + v2.m_z);
}
Vector3D operator-(const Vector3D& v1, const Vector3D& v2)
{
return Vector3D(v1.m_x - v2.m_x, v1.m_y - v2.m_y, v1.m_z - v2.m_z);
}
```
接下来是第二个问题,实现一个Student类:
```c++
class Student {
public:
Student(float score);
static float getAverageScore();
static int getCount();
private:
float m_score;
static int s_count;
static float s_total;
};
```
构造函数如下:
```c++
Student::Student(float score)
: m_score(score)
{
s_count++;
s_total += score;
}
```
静态变量的初始化:
```c++
int Student::s_count = 0;
float Student::s_total = 0.0f;
```
静态函数实现如下:
```c++
float Student::getAverageScore()
{
return s_total / s_count;
}
int Student::getCount()
{
return s_count;
}
```
这样就完成了Student类的实现。
希望我的回答能够帮到您。
阅读全文
相关推荐
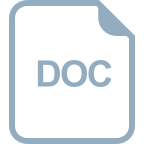
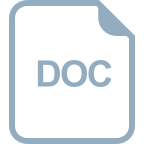
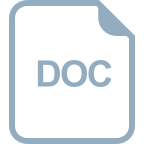




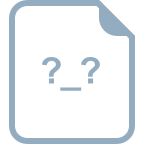
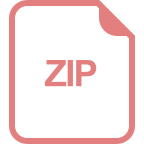
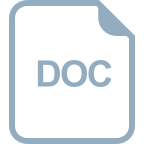
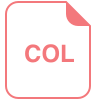






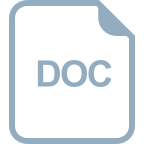