graph 和 communities 分别是 NetworkX 图和使用 fast_greedy_modularity 函数计算得到的社群列表 其中 fast_greedy_modularity 函数的代码实现
时间: 2024-05-15 17:14:23 浏览: 165
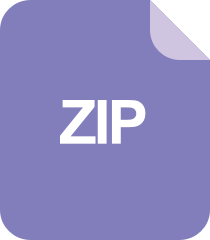
polyfit.zip_construct graph_polyfit_polyfit函数图形_曲线拟合_最小二乘法
NetworkX 是 Python 中一个常用的图论库,用于创建、操作和分析复杂网络。其中,Graph 对象是 NetworkX 中最基本的对象之一,代表着一个无向图。
以下是一个简单的使用 NetworkX 创建图的例子:
```python
import networkx as nx
# 创建一个空的无向图
G = nx.Graph()
# 添加节点和边
G.add_node(1)
G.add_edge(1, 2)
G.add_edge(1, 3)
# 绘制图形
nx.draw(G, with_labels=True)
```
fast_greedy_modularity 是一种常用的社群发现算法,它通过优化模块度(modularity)来划分网络的社群。代码实现如下:
```python
def fast_greedy_modularity(G):
# 初始化每个节点的社群为自己
communities = {node: i for i, node in enumerate(G.nodes())}
modularity = -1
while True:
# 计算当前社群划分的模块度
curr_modularity = nx.algorithms.community.modularity(G, communities)
if curr_modularity <= modularity:
break
modularity = curr_modularity
for node in G.nodes():
# 获取与当前节点相邻的所有节点
neighbors = list(G.neighbors(node))
# 计算当前节点与相邻节点的社群划分的模块度,并选择最大的社群作为当前节点的社群
best_community = communities[node]
best_modularity = curr_modularity
for neighbor in neighbors:
# 尝试将当前节点移入相邻节点所在的社群
new_communities = communities.copy()
new_communities[node] = communities[neighbor]
# 计算新的社群划分的模块度
new_modularity = nx.algorithms.community.modularity(G, new_communities)
if new_modularity > best_modularity:
best_community = new_communities[node]
best_modularity = new_modularity
# 如果最大的模块度比当前的模块度更大,则更新当前节点的社群
if best_modularity > curr_modularity:
communities[node] = best_community
# 返回社群划分结果
return list(nx.algorithms.community.label_propagation.asyn_lpa_communities(G))
```
fast_greedy_modularity 函数接受一个 NetworkX 图对象作为参数,并返回一个社群列表。函数实现中,首先初始化每个节点的社群为自己,然后不断尝试将每个节点移入与其相邻的节点的社群中,直到社群划分的模块度不再增加为止。
阅读全文
相关推荐
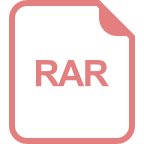

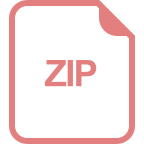
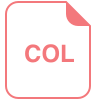
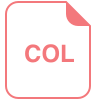
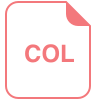
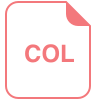
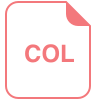
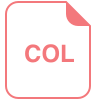
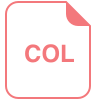
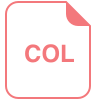
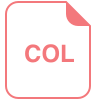
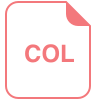
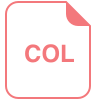



