写出基于优先队列式分支限界法设计出解决0-1背包问题的算法的代码
时间: 2023-11-26 07:04:39 浏览: 75
好的,以下是基于优先队列式分支限界法解决0-1背包问题的 Python 代码实现:
```python
import queue
class Node:
def __init__(self, level, profit, weight):
self.level = level
self.profit = profit
self.weight = weight
self.bound = 0.0
def bound(u, n, W, wt, val):
if u.weight >= W:
return 0
else:
result = u.profit
j = u.level + 1
totweight = u.weight
while (j < n and totweight + wt[j] <= W):
totweight += wt[j]
result += val[j]
j += 1
if (j < n):
result += (W - totweight) * val[j] / wt[j]
return result
def knapsack_bfs(n, W, wt, val):
q = queue.PriorityQueue()
u = Node(-1, 0, 0)
v = Node(0, 0, 0)
maxProfit = 0
u.bound = bound(u, n, W, wt, val)
q.put((-u.bound, u))
while (not q.empty()):
item = q.get()
u = item[1]
if (u.bound > maxProfit):
v.level = u.level + 1
v.weight = u.weight + wt[v.level]
v.profit = u.profit + val[v.level]
if (v.weight <= W and v.profit > maxProfit):
maxProfit = v.profit
v.bound = bound(v, n, W, wt, val)
if (v.bound > maxProfit):
q.put((-v.bound, v))
v.weight = u.weight
v.profit = u.profit
v.bound = bound(v, n, W, wt, val)
if (v.bound > maxProfit):
q.put((-v.bound, v))
return maxProfit
```
在这个算法中,我们使用了 Node 类来表示每个节点。我们使用 bound 函数来计算每个节点的界,并使用优先队列来选择具有最高界的节点进行扩展。同时,我们使用一个变量 maxProfit 来跟踪到目前为止找到的最大利润。最后,我们返回 maxProfit 作为解。
请注意,这只是一个实现示例,您可以根据自己的需求进行修改和调整。
阅读全文
相关推荐
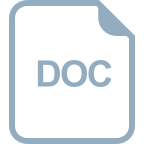
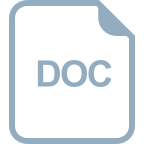
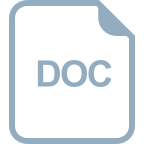














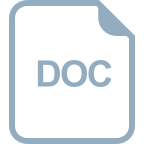
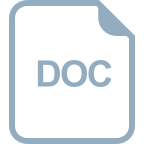