写出基于优先队列式分支限界法设计出解决0-1背包问题算法的Java语言代码
时间: 2024-03-13 18:46:11 浏览: 66
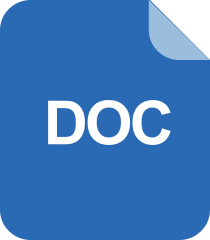
分支限界法实现0-1背包

好的,以下是基于优先队列式分支限界法解决0-1背包问题的 Java 代码实现:
```java
import java.util.PriorityQueue;
import java.util.Queue;
public class KnapsackProblem {
static class Node implements Comparable<Node> {
int level;
int profit;
int weight;
double bound;
public Node(int level, int profit, int weight) {
this.level = level;
this.profit = profit;
this.weight = weight;
}
@Override
public int compareTo(Node o) {
return Double.compare(o.bound, bound);
}
}
private static double bound(Node u, int n, int W, int[] wt, int[] val) {
if (u.weight >= W) {
return 0;
} else {
double result = u.profit;
int j = u.level + 1;
int totweight = u.weight;
while (j < n && totweight + wt[j] <= W) {
totweight += wt[j];
result += val[j];
j++;
}
if (j < n) {
result += (W - totweight) * ((double) val[j] / wt[j]);
}
return result;
}
}
public static int knapsack_bfs(int n, int W, int[] wt, int[] val) {
Queue<Node> q = new PriorityQueue<>();
Node u = new Node(-1, 0, 0);
Node v = new Node(0, 0, 0);
double maxProfit = 0;
u.bound = bound(u, n, W, wt, val);
q.offer(u);
while (!q.isEmpty()) {
u = q.poll();
if (u.bound > maxProfit) {
v.level = u.level + 1;
v.weight = u.weight + wt[v.level];
v.profit = u.profit + val[v.level];
if (v.weight <= W && v.profit > maxProfit) {
maxProfit = v.profit;
}
v.bound = bound(v, n, W, wt, val);
if (v.bound > maxProfit) {
q.offer(v);
}
v.weight = u.weight;
v.profit = u.profit;
v.bound = bound(v, n, W, wt, val);
if (v.bound > maxProfit) {
q.offer(v);
}
}
}
return (int) maxProfit;
}
public static void main(String[] args) {
int[] wt = {10, 20, 30};
int[] val = {60, 100, 120};
int W = 50;
int n = wt.length;
System.out.println("Maximum profit: " + knapsack_bfs(n, W, wt, val));
}
}
```
在这个算法中,我们使用了 Node 类来表示每个节点,并实现了 Comparable 接口以使其可以与其他节点进行比较。我们使用 bound 函数来计算每个节点的界,并使用优先队列来选择具有最高界的节点进行扩展。同时,我们使用一个变量 maxProfit 来跟踪到目前为止找到的最大利润。最后,我们返回 maxProfit 作为解。
请注意,这只是一个实现示例,您可以根据自己的需求进行修改和调整。
阅读全文
相关推荐


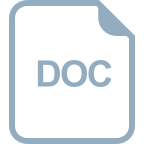




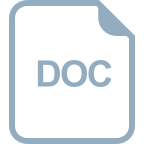
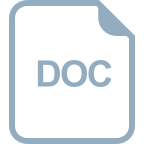






