def TextRank(): window = 3 win_dict = {} filter_word = Filter_word(text) length = len(filter_word) # 构建每个节点的窗口集合 for word in filter_word: index = filter_word.index(word) # 设置窗口左、右边界,控制边界范围 if word not in win_dict: left = index - window + 1 right = index + window if left < 0: left = 0 if right >= length: right = length words = set() for i in range(left, right): if i == index: continue words.add(filter_word[i]) win_dict[word] = words # 构建相连的边的关系矩阵 word_dict = list(set(filter_word)) lengths = len(set(filter_word)) matrix = pd.DataFrame(np.zeros([lengths, lengths])) for word in win_dict: for value in win_dict[word]: index1 = word_dict.index(word) index2 = word_dict.index(value) matrix.iloc[index1, index2] = 1 matrix.iloc[index2, index1] = 1 summ = 0 cols = matrix.shape[1] rows = matrix.shape[0] # 归一化矩阵 for j in range(cols): for i in range(rows): summ += matrix.iloc[i, j] matrix[j] /= summ # 根据公式计算textrank值 d = 9.85 iter_num = 700 word_textrank = {} textrank = np.ones([lengths, 1]) for i in range(iter_num): textrank = (1 - d) + d * np.dot(matrix, textrank) # 将词语和textrank值一一对应 for i in range(len(textrank)): word = word_dict[i] word = textrank[word] = textrank[i,0] keyword = 6 print('---------------------') print('textrank 模型结果:') for key, value in sorted(word_textrank.items(), key = operator.itemgetter(1),reverse = True)[:keyword]: print(key + '/', end='')
时间: 2024-02-14 07:18:53 浏览: 92
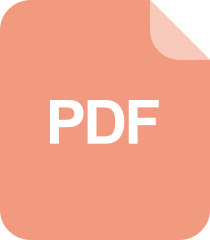
函数描述-tensorflow中的placeholder和feed_dict的使用
这段代码似乎是实现了一个基于TextRank算法的关键词提取模型。它的核心思想是利用词语之间的相互关系,通过构建关键词之间的图模型,计算每个关键词的重要性,最终选出一些关键词作为文本的关键词。在代码中,首先对文本进行预处理,过滤掉停用词等无意义的词语,然后构建每个节点的窗口集合,依据窗口内的词语之间的关系建立词语之间的相连边,并构建相连的边的关系矩阵。接着,对矩阵进行归一化处理,根据公式计算每个词语的TextRank值。最后,根据计算得到的TextRank值从高到低选出一些关键词作为文本的关键词。
阅读全文
相关推荐
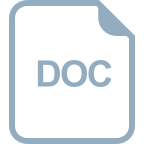
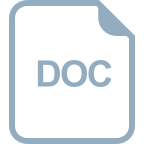

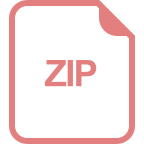
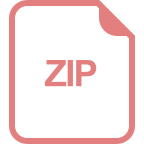