Java代码实现String算法找出两个字符串中共有的最大部分
时间: 2024-05-03 08:23:08 浏览: 95
以下是Java代码实现:
```
public class CommonSubstring {
public static String findCommonSubstring(String str1, String str2) {
int m = str1.length();
int n = str2.length();
int[][] L = new int[m + 1][n + 1];
int len = 0, row = 0, col = 0;
for (int i = 0; i <= m; i++) {
for (int j = 0; j <= n; j++) {
if (i == 0 || j == 0) {
L[i][j] = 0;
} else if (str1.charAt(i - 1) == str2.charAt(j - 1)) {
L[i][j] = L[i - 1][j - 1] + 1;
if (L[i][j] > len) {
len = L[i][j];
row = i;
col = j;
}
} else {
L[i][j] = 0;
}
}
}
if (len == 0) {
return "";
}
StringBuilder sb = new StringBuilder();
while (L[row][col] != 0) {
sb.insert(0, str1.charAt(row - 1));
row--;
col--;
}
return sb.toString();
}
public static void main(String[] args) {
String str1 = "abcdefg";
String str2 = "defghijk";
System.out.println("Two strings: " + str1 + ", " + str2);
System.out.println("Common substring: " + findCommonSubstring(str1, str2));
}
}
```
在这个示例中,我们首先定义了一个名为`findCommonSubstring()`的静态方法,它接收两个字符串`str1`和`str2`作为参数。我们使用一个二维数组`L`来存储计算出的最大公共子字符串的长度,并使用三个变量`len`、`row`和`col`来跟踪最大公共子字符串的长度、行索引和列索引。我们使用两个嵌套的for循环来计算`L`数组中的所有元素。如果当前字符匹配,则将`L[i][j]`设置为`L[i-1][j-1]+1`,并检查是否需要更新`len`、`row`和`col`。如果两个字符不匹配,则将`L[i][j]`设置为0。最后,我们检查`len`是否为0,如果是,则返回一个空字符串,否则将最大公共子字符串添加到一个StringBuilder对象中,并返回它的字符串表示形式。
在`main()`方法中,我们定义了两个字符串`str1`和`str2`,并调用`findCommonSubstring()`方法来查找它们的最大公共子字符串。最后,我们将结果打印到控制台上。
阅读全文
相关推荐
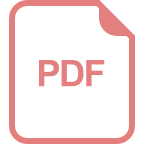
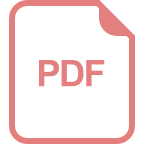
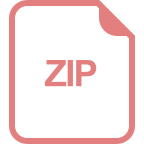















