不使用栈来编写一个算术表达式求值的c语言程序
时间: 2024-05-15 09:12:39 浏览: 158
可以使用递归来实现算术表达式求值,以下是一个示例程序:
```c
#include <stdio.h>
int eval(char *expr, int *pos) {
int num = 0;
char op = '+';
while (expr[*pos] != '\0' && op != ')') {
if (expr[*pos] >= '0' && expr[*pos] <= '9') {
num = num * 10 + (expr[*pos] - '0');
} else if (expr[*pos] == '(') {
(*pos)++;
num = eval(expr, pos);
} else if (expr[*pos] == '+' || expr[*pos] == '-' || expr[*pos] == '*' || expr[*pos] == '/') {
op = expr[*pos];
} else if (expr[*pos] == ')') {
(*pos)++;
break;
}
(*pos)++;
}
if (op == '+') {
return num;
} else if (op == '-') {
return -num;
} else if (op == '*') {
return num * eval(expr, pos);
} else if (op == '/') {
return eval(expr, pos) / num;
}
}
int main() {
char expr[100];
printf("Enter an arithmetic expression: ");
scanf("%s", expr);
int pos = 0;
int result = eval(expr, &pos);
printf("Result: %d\n", result);
return 0;
}
```
该程序读入一个算术表达式,然后使用递归进行求值。在递归函数中,我们遍历表达式,根据不同的字符进行不同的操作。如果遇到数字,我们将其加入当前数字中;如果遇到左括号,我们递归地求解括号内的表达式;如果遇到运算符或右括号,我们根据前面的运算符进行相应的计算。最终,我们返回计算结果。
需要注意的是,这个程序没有进行任何错误检查,所以不保证在所有情况下都能正确求解表达式。
阅读全文
相关推荐
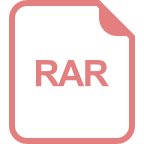












