1.设计哈夫曼树。具体构造方法如下:以字符集{A,B,C,D,E,F,G,H}作为叶子结点,以各个字符出现的字符次数{5,29,7,8,14,23,3,11}作为各个叶子结点的权值构造哈夫曼树。 2.设计哈夫曼编码。按照构造出来的哈夫曼树,规定哈夫曼树的左分支为0,右分支为1,则从根结点到每个叶子结点所经过的分支对应的0和1组成的序列便是该结点对应字符的哈夫曼编码。使用c语言实现
时间: 2024-01-18 10:03:22 浏览: 90
好的,下面是一个用C语言实现的哈夫曼树和哈夫曼编码的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 哈夫曼树结构体
typedef struct node {
int weight; // 权值
char value; // 值
struct node *left; // 左子树
struct node *right; // 右子树
} Node;
// 哈夫曼编码结构体
typedef struct code {
char value; // 值
char *code; // 编码
} Code;
// 构造哈夫曼树
Node *huffman_tree(int weights[], char values[], int n) {
Node *nodes[n];
for (int i = 0; i < n; i++) {
nodes[i] = (Node *)malloc(sizeof(Node));
nodes[i]->weight = weights[i];
nodes[i]->value = values[i];
nodes[i]->left = NULL;
nodes[i]->right = NULL;
}
while (n > 1) {
int min1 = 0, min2 = 1;
if (nodes[min1]->weight > nodes[min2]->weight) {
int temp = min1;
min1 = min2;
min2 = temp;
}
for (int i = 2; i < n; i++) {
if (nodes[i]->weight < nodes[min1]->weight) {
min2 = min1;
min1 = i;
} else if (nodes[i]->weight < nodes[min2]->weight) {
min2 = i;
}
}
Node *parent = (Node *)malloc(sizeof(Node));
parent->weight = nodes[min1]->weight + nodes[min2]->weight;
parent->value = 0;
parent->left = nodes[min1];
parent->right = nodes[min2];
nodes[min1] = parent;
nodes[min2] = nodes[n-1];
n--;
}
return nodes[0];
}
// 生成哈夫曼编码
void huffman_encoding(Node *node, char *code, int depth, Code *codes, int *index) {
if (node->left == NULL && node->right == NULL) {
Code c;
c.value = node->value;
c.code = (char *)malloc(sizeof(char) * (depth+1));
strcpy(c.code, code);
codes[*index] = c;
(*index)++;
} else {
code[depth] = '0';
huffman_encoding(node->left, code, depth+1, codes, index);
code[depth] = '1';
huffman_encoding(node->right, code, depth+1, codes, index);
}
}
// 比较函数,用于排序
int compare_codes(const void *a, const void *b) {
Code *ca = (Code *)a;
Code *cb = (Code *)b;
return ca->value - cb->value;
}
// 测试函数
int main() {
int weights[] = {5, 29, 7, 8, 14, 23, 3, 11};
char values[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'};
int n = sizeof(weights) / sizeof(weights[0]);
Node *root = huffman_tree(weights, values, n);
Code codes[n];
int index = 0;
char code[n];
huffman_encoding(root, code, 0, codes, &index);
qsort(codes, n, sizeof(Code), compare_codes);
printf("字符集:");
for (int i = 0; i < n; i++) {
printf("%c ", codes[i].value);
}
printf("\n");
printf("权值:");
for (int i = 0; i < n; i++) {
printf("%d ", weights[i]);
}
printf("\n");
printf("哈夫曼编码:");
for (int i = 0; i < n; i++) {
printf("%c:%s ", codes[i].value, codes[i].code);
}
printf("\n");
return 0;
}
```
这个代码实现了一个哈夫曼树和哈夫曼编码的生成过程。首先定义了一个Node结构体来表示哈夫曼树的节点,其中包括节点权值、节点值、左子树和右子树。然后通过huffman_tree函数构造哈夫曼树,该函数接收权值和值以及它们的个数作为输入,返回哈夫曼树的根节点。接着通过huffman_encoding函数生成哈夫曼编码,该函数接收当前节点、当前的编码、编码深度、存储编码和编码的个数的数组和下标作为输入,返回包含所有字符对应哈夫曼编码的数组。最后通过qsort函数对编码数组进行排序,然后通过打印输出的方式展示字符集、权值和哈夫曼编码。
阅读全文
相关推荐
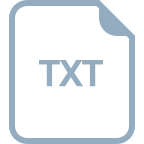




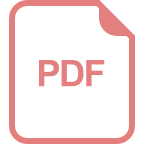
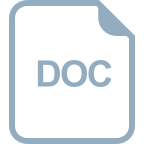
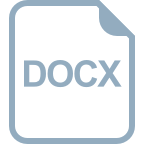
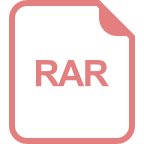
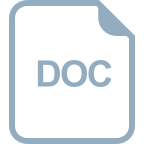
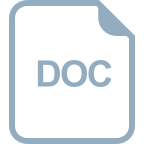
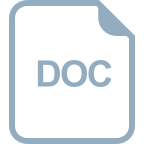
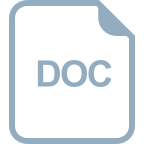
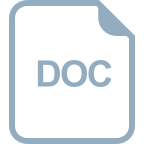
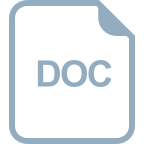
