int main() { int x,y,c,z; Stack *S; setNULL(S);
时间: 2024-05-05 15:16:52 浏览: 19
As an AI language model, I can only provide suggestions on how to improve the code you posted. Here are a few suggestions:
1. Declare the data type of the Stack variable. For example, if your Stack implementation is based on an array, you could declare it as follows:
```c++
int main() {
int x, y, c, z;
int maxSize = 100; // Maximum size of the stack
int top = -1; // Initialize the top pointer to -1
int* S = new int[maxSize]; // Allocate memory for the stack
// ...
}
```
2. Initialize the Stack before using it. You could define a function to initialize the Stack as follows:
```c++
void initStack(int* S, int& top) {
top = -1;
}
```
Then call this function before using the Stack:
```c++
int main() {
int x, y, c, z;
int maxSize = 100; // Maximum size of the stack
int top = -1; // Initialize the top pointer to -1
int* S = new int[maxSize]; // Allocate memory for the stack
initStack(S, top); // Initialize the stack
// ...
}
```
3. Check if the Stack is empty before popping an element. You could define a function to check if the Stack is empty as follows:
```c++
bool isEmpty(int top) {
return top == -1;
}
```
Then use this function to check if the Stack is empty before popping an element:
```c++
int main() {
int x, y, c, z;
int maxSize = 100; // Maximum size of the stack
int top = -1; // Initialize the top pointer to -1
int* S = new int[maxSize]; // Allocate memory for the stack
initStack(S, top); // Initialize the stack
// ...
if (!isEmpty(top)) {
int element = S[top];
top--;
// ...
}
// ...
}
```
I hope this helps! Let me know if you have any further questions.
相关推荐
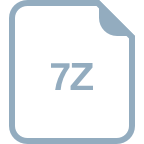
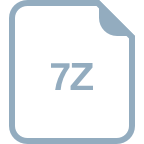















